Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial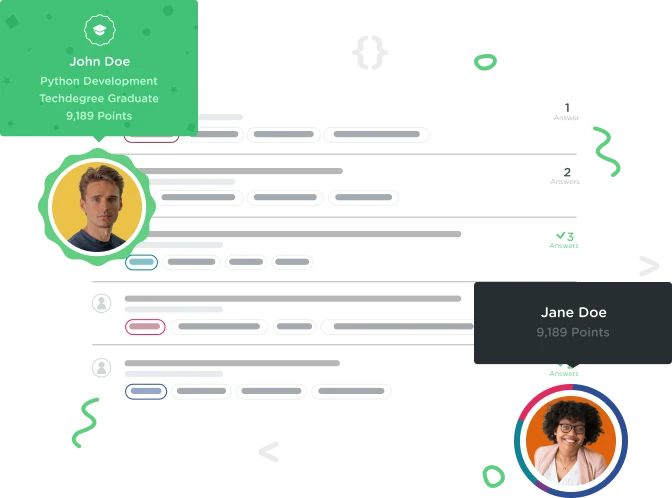

akhilesh Bhartiya
1,048 PointsCreate a function named nchoices() that takes an iterable and an integer. The function should return a list of n random
help
import random
def nchoices(iterable, interger):
my_list = []
for index, value in enumerate(iterable):
my_list.append(value)
return random.choice(my_list),interger
4 Answers
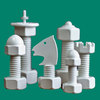
Steven Parker
231,275 PointsHere are a few hints that may help:
- you shouldn't have to enumerate the iterable, you just need to pick from it
- the last line has a typo: the parentheses enclose only the first item instead of all the arguments
- be sure to build and return a list of random choices, don't just return one choice from the list
Here's a potentially even better hint: There might be an easier way to perform this task. Check out the Python documentation for random and look at the method named sample. Would it be useful here?
And were you aware that there is an updated version of the Python Collections course?

akhilesh Bhartiya
1,048 Pointsimport random
def nchoices(iterable, integer):
listi = []
for item in iterable:
listi.append([item, iterable])
return random.choice(listi)

akhilesh Bhartiya
1,048 Pointsany help please I don't understand the question

Iain Simmons
Treehouse Moderator 32,305 PointsFor this, it helps to break it down to what your inputs are, how they should be used/interact with each other and what your expected output is.
Inputs:
- iterable of any length, with any type(s) of items, from which to randomly select items (including duplicates).
- positive integer for the number of random items to return
How they are used:
- loop for
n
iterations/times, wheren
is the integer passed in - for each iteration of the loop, randomly select an item from the iterable
Expected output:
- The randomly selected items as a new list (would need a list inside the function to store them in) with length equal to the integer passed in
I don't want to just give you the answer, because then you don't really learn anything, but you will need the following functions/methods:
And there's also a new method in Python 3.6 called random.choices that does exactly what this challenge is asking for, but this course was made well before this was available.

Sneha Nagpaul
10,124 Pointsexample input : [1, 20, 3, 9, 100] , 2 example output : [20, 9]
or
I/p : "abcdefg", 2 o/p: ['c','g']
Now, you want to make sure if the questions asks for choosing with or without replacement. In the above examples, I am assuming without replacement i.e. the same item cannot be drawn again. This can also be seen as drawing a sample from the collection. For the built in function in the random library, use Steven's suggestion and look up random.sample().
Or, you could write your own--- pick something, remove, repeat until enough choices have been made. Or maybe, pick some random numbers from the indices of the iterable and return those values.
From looking at the code you have posted, you should consider the length of the loop. Do you want to see every value in the iterable? How many values do you want to see? (Does it matter if I see [1, 3, 100] from example 1?)That's how long the loop should be.
Hope this helped.
akhilesh Bhartiya
1,048 Pointsakhilesh Bhartiya
1,048 PointsNot helpful at all