Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial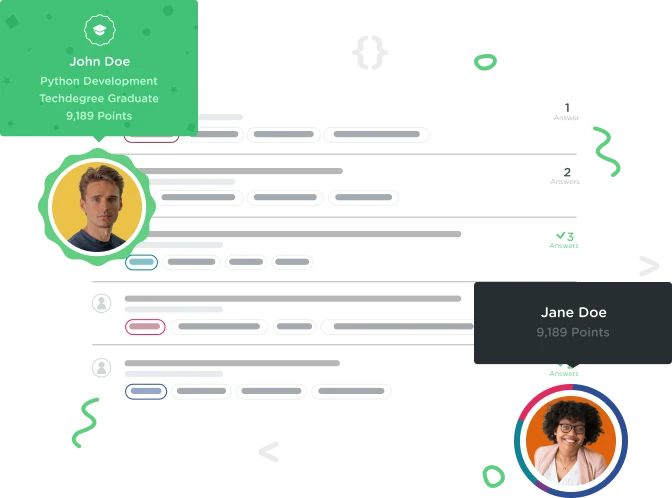

frankgusto
4,091 PointsCreate a function named string_factory that accepts a list of dictionaries and a string. Return a new list using .format
Create a function named string_factory that accepts a list of dictionaries and a string. Return a new list build by using .format() on the string, filled in by each of the dictionaries in the list.
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
# create the function string_factory
# extract each dictionary from the list - dicts[0] = {'name': 'Michelangelo', 'food': 'PIZZA'}
# fill the string using "**" trick
# put each string into the new list
new_list = []
def string_factory():
# I could run for in loop, but I don't know how to do string.format one each member of the list (dicts[0])
# for each_dict in dicts:
new_dicts1 = dicts[0]
new_dicts2 = dicts[1]
new_dicts3 = dicts[2]
new_dicts4 = dicts[3]
# how to run string.format on each member of dicts list ???
new_string1 = string.format(**new_dicts1)
new_string2 = string.format(**new_dicts2)
new_string3 = string.format(**new_dicts2)
new_string4 = string.format(**new_dicts2)
# is there a way to append / add all new strings at the same time ?
new_list.append(new_string1)
new_list.append(new_string2)
new_list.append(new_string3)
new_list.append(new_string4)
return new_list
[MOD: added ```python markdown formatting -cf]
3 Answers
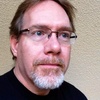
Chris Freeman
Treehouse Moderator 68,454 PointsYour code is very close!
- add missing parameters for dicts and the string
- remove inner loop. You can expand directly from the dict:
new_list = []
def string_factory(dicts, string): # <-- Added parameters
for each_dict in dicts:
# remove inner loop
# for key in each_dict: # <-- I am struggling with running second for in loop for each dictionary in the list
new_string = string.format(**each_dict) # <-- replace 'key' with 'each_dict'
new_list.append(new_string)
return new_list
This code will pass. Indentation should be cleaned up after edits.

Ryan Flyod
1,939 PointsHi Chris,
I've been stuck on this problem for a while now. I ended up resorting to copy and pasting your code. However, it still didn't pass. I pasted EXACTLY what you had, and it returns:
Bummer! string_factory() missing 1 required positional argument: 'string'
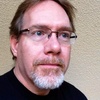
Chris Freeman
Treehouse Moderator 68,454 PointsHi Ryan, this solution was intended for a retired Python course that has been replaced with updated material and challenges.
The old challenge passed in the template string as an argument. The new challenge expects you to refer to the global variable template
To modify the above solution for the new challenge:
- remove
string
parameter fromstring_factory()
definition - replace
string
reference withtemplate
This should pass the new challenge.

frankgusto
4,091 PointsBasically it could look like something like this... I am struggling with running second for in loop for each dictionary in the list. Please help.
new_list = []
def string_factory():
for each_dict in dicts:
for key in each_dict: # <-- I am struggling with running second for in loop for each dictionary in the list
new_string = string.format(**key)
new_list.append(new_string)
return new_list
[MOD: added ```python markdown formatting -cf]

frankgusto
4,091 PointsChris, thanks again!
Ryan Flyod
1,939 PointsRyan Flyod
1,939 PointsChris,
Thanks so much, that worked. This was the first time I was really stumped, so I appreciate it a lot. Thanks.