Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial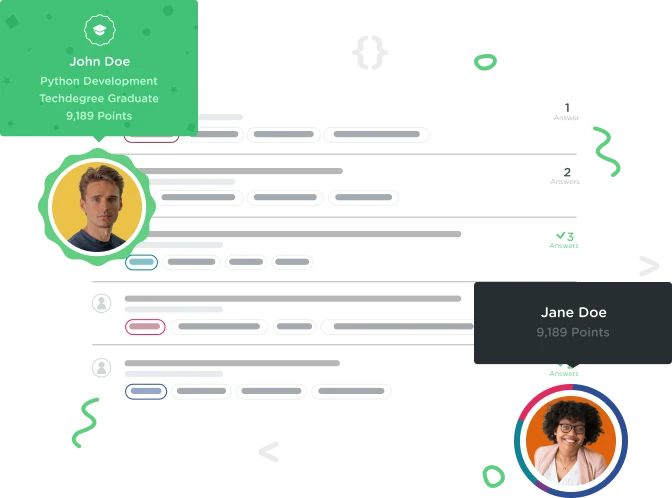

anhedonicblonde
3,133 PointsCreate a function named word_count...
I'm doing a review of my Python studies and finding the parts where I didn't get things the first time around. I'm having trouble seeing what is wrong with my code for the word_count challenge - can someone tell me where I have gone wrong? Thanks so much!
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(string):
my_string = string.lower()
word_list = my_string.split()
word_dict = {}
count = 0
for word in word_list:
if word in word_dict:
count +=1
else:
word_dict[word] = 1
return count
3 Answers
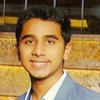
Anish Walawalkar
8,534 PointsHey, you're doing great, you just made a few small mistakes:
- the question does not ask you to lowercase the string, so you do not need to do it
- you should check using word in word_dict.keys() thought just checking word in word_dict is not wrong
- The question asks you to return a dictionary mapping words to their count not an overall count
here is the code:
def word_count(string):
word_list = string.split()
word_dict = {}
for word in word_list:
if word in word_dict.keys():
word_dict[word] +=1
else:
word_dict[word] = 1
return word_dict
Hope this helps

Andrey Chernykh
Courses Plus Student 2,552 PointsEasier way would be count()
The method count() returns count of how many times obj occurs in list.
def word_count(string):
my_string = string.lower()
word_list = my_string.split()
word_dict = {}
for word in word_list:
word_dict[word]=word_list.count(word)
return word_dict

Andrey Chernykh
Courses Plus Student 2,552 PointsJust a small addition , there is no need of using .keys() in this case , try to go easy and with less code

anhedonicblonde
3,133 PointsThanks for your answer!
anhedonicblonde
3,133 Pointsanhedonicblonde
3,133 PointsOh, cool! Thank you so much!! I really appreciate it- I understand now, thank you :)