Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial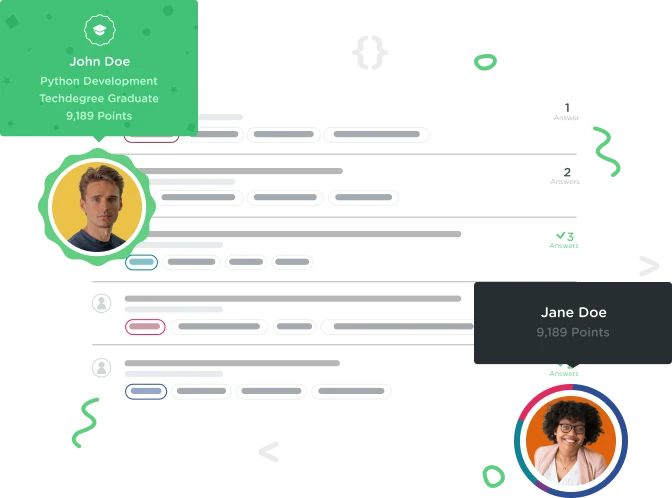
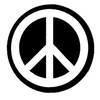
john larson
16,594 PointsCreate a function named word_count() that takes a string. Return a dictionary with each word in the string as the key...
Create a function named word_count() that takes a string. Return a dictionary with each word in the string as the key and the number of times it appears as the value.
- Bummer! Didn't get the right count for some of the words.
- I've tested it with several different strings and it appears to work
- what am I missing?
# E.g. word_count("I am that I am") gets back a dictionary like:
# {'i': 2, 'am': 2, 'that': 1}
# Lowercase the string to make it easier.
# Using .split() on the sentence will give you a list of words.
# In a for loop of that list, you'll have a word that you can
# check for inclusion in the dict (with "if word in dict"-style syntax).
# Or add it to the dict with something like word_dict[word] = 1.
def word_count(s):
s = s.lower()
dct_cnt = {}
count = 0
list_s = s.split()
for word in list_s:
if word in dct_cnt:
count +=1
dct_cnt.update({word: count})
else:
count = 1
dct_cnt.update({word: count})
return dct_cnt
1 Answer

Cheo R
37,150 PointsHello John, you're on the right track.
Your code fails with the test string:
test = "This test string is only a test string"
word_count(test)
{'this': 1, 'is': 1, 'test': 2, 'string': 3, 'a': 1, 'only': 1}
Because it thinks the word string has been seen three times. Here is a chart showing word and count:
WORD----- | ----IN DICT---- | ----COUNT |
---|---|---|
this | No | 1 |
test | no | 1 |
string | no | 1 |
this | YES | 2 |
is | no | 1 |
only | No | 1 |
a | no | 1 |
test | YES | 2 |
string | YES | 3 |
The last three words are important. Because a has not been found in the dictionary, count is one. count is still one, so when test is found in the dictionary, count increases by one. Now, before it checks if string is in the dictionary, count is two, so when it finds string, it increases by one.
To fix your problem, you can either get the word's value (since you already know it's in the dictionary) and update it, like:
if word in dct_cnt:
count = dct_cnt[word]
count +=1
dct_cnt.update({word: count})
test = "This test string is only a test string"
word_count(test)
{'this': 1, 'is': 1, 'test': 2, 'string': 2, 'a': 1, 'only': 1}
or combine them into one line:
if word in dct_cnt:
dct_cnt[word] += 1
test = "This test string is only a test string"
word_count(test)
{'this': 1, 'is': 1, 'test': 2, 'string': 2, 'a': 1, 'only': 1}
I'm sure there are other ways of doing it, but I'm tired.
john larson
16,594 Pointsjohn larson
16,594 PointsCheo, thanks for your response. Nicely presented