Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial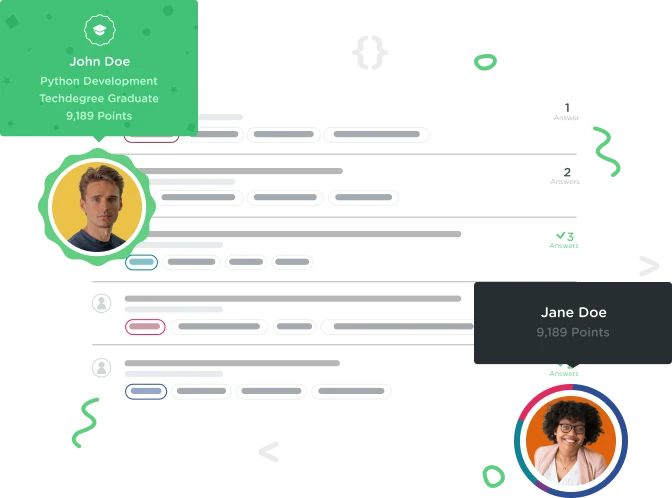

Jose Coto
11,466 PointsCreate a function that calculates the difference between dates and checks if it is bigger than a number
Hi, I'm having troubles solving this one. I cannot get how to compute the difference between dates. I suspect there is an easier way that the one I try below. Your help will be greatly appreciated.
import datetime
birthdays = [
datetime.datetime(2012, 4, 29),
datetime.datetime(2006, 8, 9),
datetime.datetime(1978, 5, 16),
datetime.datetime(1981, 8, 15),
datetime.datetime(2001, 7, 4),
datetime.datetime(1999, 12, 30)
]
is over_13(date):
today = datetime.datetime.today()
diff = today - date
return round(diff.seconds/86400) >= 4745
1 Answer
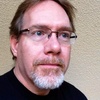
Chris Freeman
Treehouse Moderator 68,457 PointsHi Jose, two issues with your current code: the function definition syntax, and the use of the timedelta object diff
The proper function should be defined using the keyword def
and no spaces in the function name:
def is_under_13(date):
Subtracting twodaatetime
objects results in a timedelta
object. In your code, you've call it diff
. A timedelta
object has these properties (from help(datetime.timedelta
):
| days
| Number of days.
|
| microseconds
| Number of microseconds (>= 0 and less than 1 second).
|
| seconds
| Number of seconds (>= 0 and less than 1 day).
Note that the seconds
attribute contains only the partial seconds of less than 1 day. You need to use the days
attribute. Add this to your code:
def is_over_13(date):
#today = datetime.datetime.today() #<-- not needed defined as global
diff = today - date
return diff.days >= 4745