Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial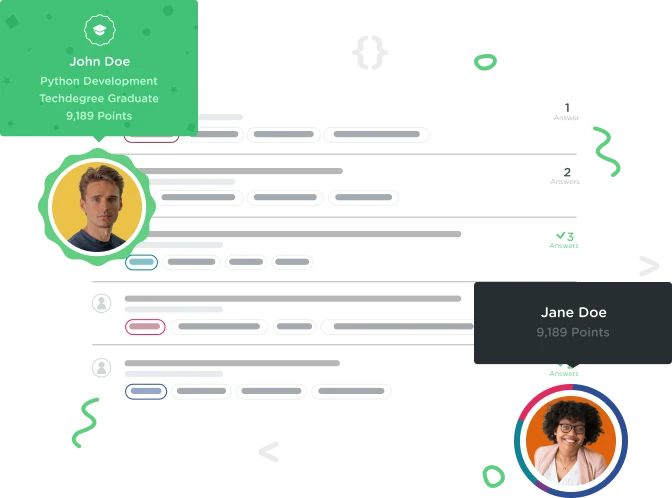
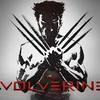
Wolverine .py
2,513 Pointscreate a function that will return a string which has a dictionary added at the end
the list i have created doesn't have the correct output
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string):
new_list = ()
new_list = list("Hi, I'm {} and I love to eat {}! ".format("Michelangelo, Garfield, Walter, Galactus", "PIZZA, lasanga, pancakes, worlds"))
return new_list
2 Answers
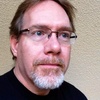
Chris Freeman
Treehouse Moderator 68,454 PointsYour code is missing some key elements:
def string_factory(dicts, string):
# Start with Blank list
new_list = []
#loop over each dictionary listed in "dicts"
for each_dict in dicts:
# the string variable uses named fields.
# populate these named fields in string with the values using the values in each_dict
outstring = string.format(name=each_dict['name'], food=each_dict['food'])
# append new outstring to new_list
new_list.append(outstring)
# return completed new_list
return new_list
These statements can be compress to:
def string_factory(dicts, string):
new_list = []
for each_dict in dicts:
new_list.append(string.format(name=each_dict['name'], food=each_dict['food']))
return new_list
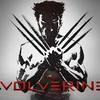
Wolverine .py
2,513 PointsThank you Chris and Jason, i had forgotten the looping. I will practice more.
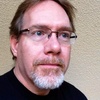
Chris Freeman
Treehouse Moderator 68,454 PointsI wanted to comment about your question title. If by "a string with a dictionary at the end" you mean the curly brackets inside the string, those not there to represent a dictionary.
The use of {}
in python syntax has multiple meanings. The main one as you mention is to indicate a dictionary. Additionally,{}
are used in regular expression syntax, and as in this case they are used as fields
for the str.format()
method.
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsIt would be easier to unpack the dictionary that you have especially if you had more keys.
new_list.append(string.format(**each_dict))
Chris Freeman
Treehouse Moderator 68,454 PointsChris Freeman
Treehouse Moderator 68,454 PointsAgreed. Sometimes the
**
notation can be overwhelming or muddle the key concepts being taught for newer students. There is also the caveats for using unpacking, like in this case, what if theeach_dict
had more than the two keys needed for the format: