Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial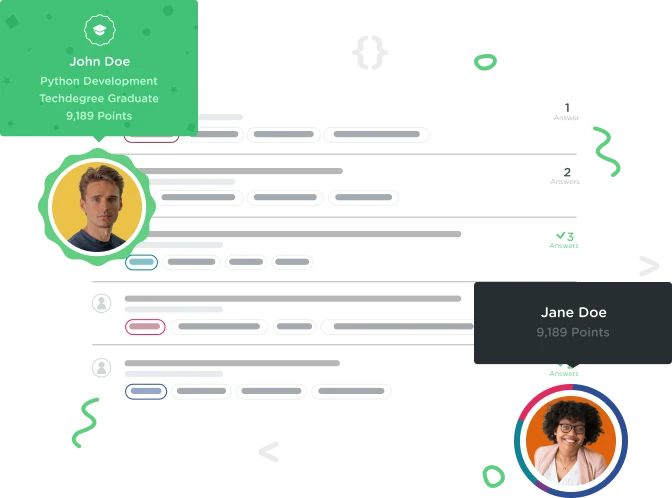

Shubham Modi
5,799 PointsCreate a helper method that returns whether or not the GoKart needs to be charged. Make it public and name it isBatteryE
How do I do it??
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
public Integer isBatteryEmpty( ) {
return mBarsCount;
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
}
3 Answers
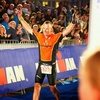
Steve Hunter
57,712 PointsHi there,
You're along the right lines.
First, the helper method will return a true
or false
in answer to the question, "is the battery empty".
To do that, compare mBarsCount
to zero like mBarsCount == 0
and return that. We use the double equals to test whether the two things are the same.
So, you want to amend your method to return a boolean
not an Integer
and then return the above comparison. There's just one line required inside your method; the result of the comparison being returned to the caller.
To summarise: Create a public method that returns a boolean and takes no parameters; then return the comparison between mBarsCount
and zero. Job done!
Let me know how you get on.
Steve.
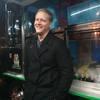
gregory gordon
Full Stack JavaScript Techdegree Student 14,652 Pointspublic boolean isBatteryEmpty( ) {
if (mBarsCount <= 0){
return true;
}
return false;
}
how does this work why is the return false not in the bracets or need anything else declaired?
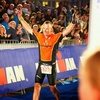
Steve Hunter
57,712 PointsHi Gregory,
Your code looks fine and it should work, yes. It could be more brief, as we'll get to in a minute.
You are testing a binary situation; there are two outcomes - either mBarsCount
is less than or equal to zero or it is not. There's no middle ground; no overlap at all. mBarsCount
is in one camp or the other.
So, your if statement is checking which camp mBarsCount
has put its tent in. If it is zero or less, the value true
is returned. At this point, your if
statement ends. If the code execution has reached this far, that is because the initial condition was not met. So, mBarsCount
is greater than zero - there is no alternative. Had mBarsCount
been zero or less, the method would have exited at return true
. So, now we've got beyond the if
statement, it is safe to just return false
as there's no other possible outcome to handle.
Do we need the if
statement? No, we don't. We can just return the evaluation of the comparison. That comparison produces the same true
or false
outcome without needing to stipulate what to return.
public boolean isBatteryEmpty(){
return mBarsCount == 0;
}
You can use less than or equal to, or just ==`` for this - that's a question of how often you test and what values you decrement. Logically, however, your code should not allow a battery to be less than empty. That makes no sense! The above method compares the value of
mBarsCountto zero and returns whether they are equal (
true) or not (
false```).
I hope that helps,
Steve.
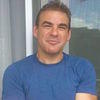
John Miller
4,007 PointsShubham, Look at the following code with comments:
public class GoKart {
public static final int MAX_BARS = 8;
private String mColor;
private int mBarsCount;
public GoKart(String color) {
mColor = color;
mBarsCount = 0;
}
// It looks like you are on the right track with this method, but lets add to it bit
public boolean isBatteryE( ) { // Change name to match requirement (instructions)
// Check Battery to see if it needs to be charged
if (mBarsCount <= 0){
return true; // If mBarsCount is less than or equals 0,
//return boolean "true" - the battery needs to be charged
}
return false; // If mBarsCount is not less than or equal to 0,
// return boolean 'false' the battery does not need to be charged
}
public String getColor() {
return mColor;
}
public void charge() {
mBarsCount = MAX_BARS;
}
}
Look at this code and see if it makes sense. Your code doesn't have to be the exact same, just check the logic and syntax
If you have more questions, feel free to reply to request for more information.
Cheers and happy coding,