Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial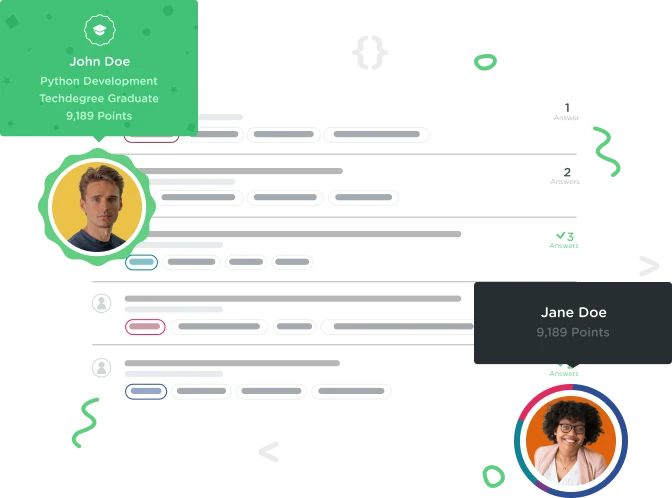

Zan Le
1,016 PointsCreate a list of tuples
Hi, could you kindly help why does this code pass please. It appears to produce the expected output.
many thanks
# combo([1, 2, 3], 'abc')
# Output:
# [(1, 'a'), (2, 'b'), (3, 'c')]
def combo(var1, var2):
list_1 = list(var1)
list_2 = list(var2)
return zip(list_1, list_2)
3 Answers

Zan Le
1,016 PointsThank Edwin Carrasquillo . So I rewrite the function, unpack the tuple and add the * in the argument to accept more than 1 argument as per below. However, it still does not pass. Could you kindly help to have a look please. thank u
def combo(*var): a,b = var list_1 = list(a) list_2 = list(b) return zip(list_1, list_2)

Edwin Carrasquillo
10,315 PointsI don't just want to give you the answer because you won't really learn that way. My advice was to push you in the right direction. I am always willing to help a fellow pythoner.
Your code below is creating variables of the contents of the unpacking. Then using the 'zip()' function which yes will work, but very much like other functions (i.e append() & extend()) 'zip()' will return nothing. Try putting your wrapper zip() in a variable and then returning your variable.
def combo(*var):
a,b = var
list_1 = list(a)
list_2 = list(b)
results = zip(list_1, list_2)
return results
If you want I would recommend to try to use the examples from the video you watched before this challenge. You will be using a combination of tuples, enumerate, and a for loop to iterate through the contents of each part of the separated tuples (a, b).
Pro-tip: the enumerate() function will essentially do what was the zip() function does for you. I know zip() makes it easy, but sometimes its good to go the long way around.
Also try to use the markdown for any other replies. It will help me read your code better. The "Markdown Cheatsheet" is clickable and in bold below the comment box.

Zan Le
1,016 PointsThanks Edwin!
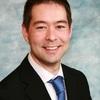
Mark Chesney
11,747 PointsFYI for anyone reading this: the code in the original question worked just fine when I ran the following function call:
list(combo([1, 2, 3], 'abc'))
Edwin Carrasquillo
10,315 PointsEdwin Carrasquillo
10,315 PointsIt seems that you are trying to approach this as if you are receiving two separate arguments. In this scenario you are receiving a tuple that contains two arguments. You will have to unpack the tuple and your argument needs the '*' on it. It will be excepting more then 1 argument.
Using variables:
a,b = var # Which means a=[1,2,3] and b='abc'