Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial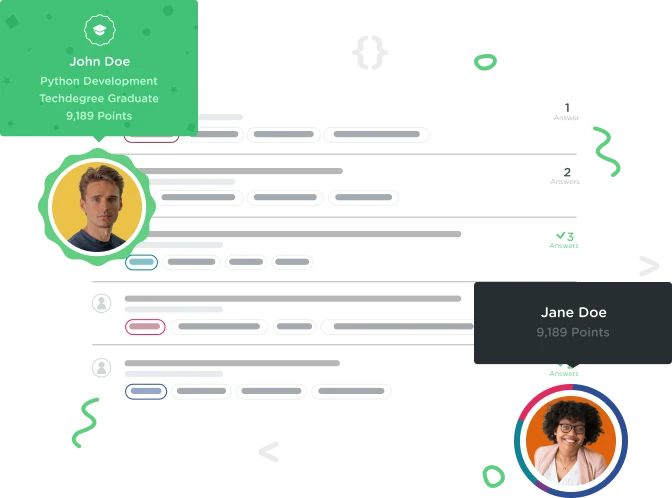
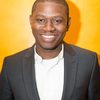
Welby Obeng
20,340 PointsCreate a macro named hide_email. It should take a User as an argument. Print out the email attribute of the User in the
Can someone help with this?
from flask import Flask, render_template
app = Flask(__name__)
@app.route('/')
def index():
class User:
email = None
user = User()
user.email = 'kenneth@teamtreehouse.com'
return render_template('user.html', user=user)
{% macro hide_email(User.email) %}
{{total = len(User.email)}}
{{position_at = User.email.index('@')}}
{{ll = []}}
{% for i,l in enumerate(User.email) %}
{% if i > 0 and i < position_at: %}
{% ll.append('*') %}
{% else: %}
{% ll.append(l) %}
{{''.join(ll)) }}
{% endif %}
{% endfor %}
1 Answer

Iain Simmons
Treehouse Moderator 32,305 PointsSo first up, the challenge states that you should "take a User
as an argument", so you don't need the .email
in the macro expression. You do need to access the email
attribute of the User
that is passed in, elsewhere in your code (as you have done).
Secondly, we haven't covered setting variables in Jinja/Flask templates in the videos, and so that isn't the solution here (though it is possible with a different syntax). Instead you'll need to call the list methods inline, rather than assigning to a variable and using the variable later.
Third, enumerate
can't be used in Jinja/Flask templates. They do have special variables for accessing the loop index, but we also haven't covered those.
This challenge can instead be completed using a for
loop and list slices (e.g. list[1:5]
). You can use the index
method to find the position of the @
symbol to use in the slices, or use the split
method as is suggested in the challenge.
This is certainly one of the trickier challenges, so perhaps try put together your own Python script (not Jinja/Flask template) in another file, using the 'print' method to output to the console (with the end
parameter set to a blank string so you don't get a new line in between printed characters), and run it to see the results of your loops and slices. For example:
class User:
email = None
user = User()
user.email = 'kenneth@teamtreehouse.com'
# only change the code in the for loop below:
for c in user.email:
print(c, end='')
Good luck and let us know how you go or if you need more help!