Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial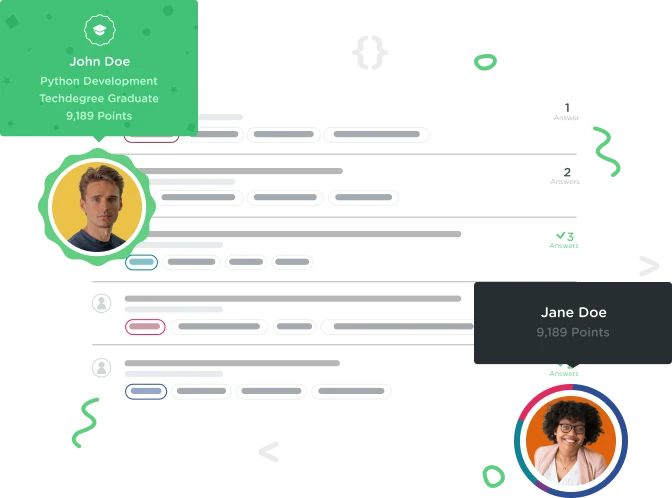
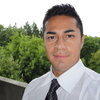
Walter Cortez
4,071 PointsCreate a max function task 1 of 2.
need help with this..
what is wrong here?
function max(number1, number2) { if (3 > 2) { return number1;
} } max("3, 2");
1 Answer
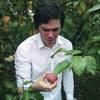
Leslie Heinzen
26,662 PointsHi Walter,
The if statement has an expression, the code inside the parentheses, which will evaluate as a Boolean (true or false). First, you need to reference the parameters of your function in your logic, instead of actual integers, like so:
if (number1 > number2) {
return number1;
}
If you used your version, (3 > 2), it will always be true regardless of which arguments are passed into the function, and so the function would always return number1 at that moment.
Next, you need to establish logic for what happens if number2 is larger than number1, because right now your function does not return anything for this case. There are a couple of ways you could do this, but the simplest way is to add an else statement:
else {
return number2;
}
Now, the function will return number2 if it is equal to or greater than number1.
The final code could look something like this:
function max(number1, number2) {
if (number1 > number2) {
return number1;
} else {
return number2;
}
}
Hope this clarifies things for you. Good luck!
EDIT: Just to further clarify, when you call the function with numbers (For example: max(2, 3)), be sure to pass in numbers, not a string, so do not include quotation marks, or the function will not evaluate correctly.