Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial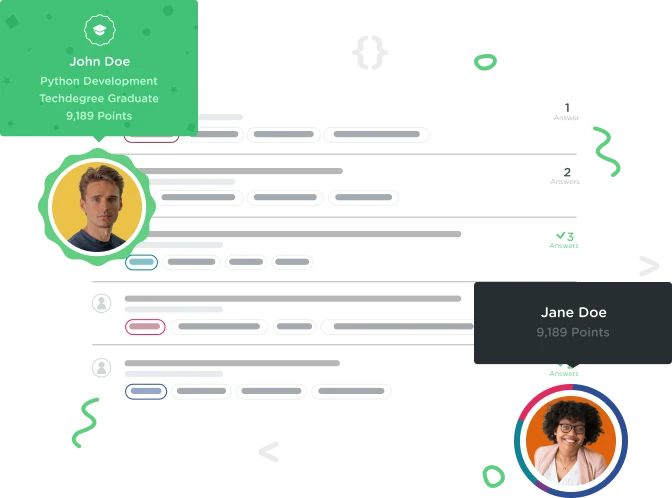
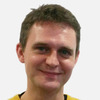
Joel Brennan
18,300 PointsCreate a method named WordsWithCountGreaterThan that takes an integer as a parameter.
Hi,
Can't get my head around this, not sure if I should even be using Linq as that has not been covered yet.
Can someone help please?
Thanks
using System.Collections.Generic;
using System.Linq;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
int currentCount;
WordCount.TryGetValue(word, out currentCount);
WordCount[word] = currentCount + 1;
}
public Dictionary<string, int> WordsWithCountGreaterThan(int i)
{
WordCount = WordCount.Where(p => p.Key.Length >= i)
.ToDictionary(p => p.Key, p => p.Value);
return WordCount;
}
}
}
2 Answers

andren
28,558 PointsYou can solve this challenge without using Linq by using a foreach loop like this:
public Dictionary<string, int> WordsWithCountGreaterThan(int i)
{
Dictionary<string, int> biggerWords = new Dictionary<string, int>();
foreach(var word in WordCount.Keys) {
if(WordCount[word] > i) {
biggerWords.Add(word, WordCount[word]);
}
}
return biggerWords;
}
However you can also solve it using Linq, and your code is almost correct. There are only two issues both of which are found in the first line of the Linq expression:
p => p.Key.Length >= i
The first issue is that you seem to have slightly misunderstood what the challenge is asking for. It wants you to return words with a count greater than i, not words that have a length greater than i. And that also touches on the second issue. The challenge specially asks for words with a count greater than i, not greater or equal to i, which is what you are checking for.
If you fix those two issues you end up with this:
public Dictionary<string, int> WordsWithCountGreaterThan(int i)
{
WordCount = WordCount.Where(p => p.Value > i)
.ToDictionary(p => p.Key, p => p.Value);
return WordCount;
}
Which will allow you to complete the challenge.

Kevin Gates
15,053 PointsThis works:
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
try
{
WordCount.Add(word, 1);
}
catch
{
WordCount[word] +=1;
}
}
public Dictionary<string,int> WordsWithCountGreaterThan(int count)
{
var resultSet = new Dictionary<string,int>();
foreach(KeyValuePair<string, int> entry in WordCount)
{
if ( entry.Value > count)
{
resultSet.Add(entry.Key,entry.Value);
}
}
return resultSet;
}
}
}
Joel Brennan
18,300 PointsJoel Brennan
18,300 PointsHi
I was indeed misundertanding the question, in particular the part: 'number of times';
I managed to get the result using the foreach loop.
Thank you for your help