Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial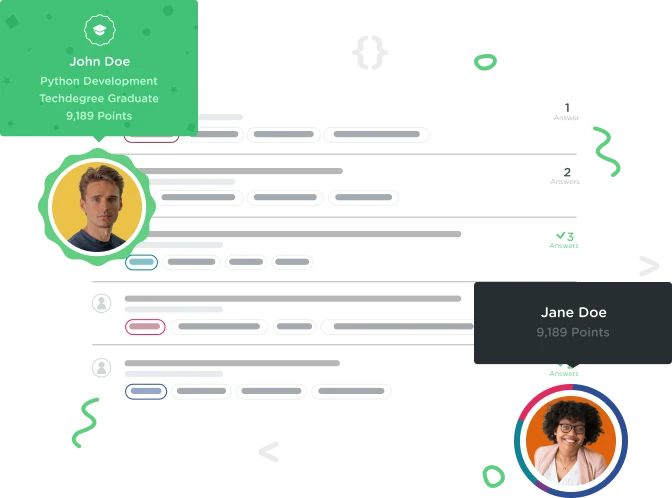

MUZ141058 Loice Kajawu
3,364 PointsCreate a method on Fish named getInfo that takes no parameter and returns a string that includes the common_name, flavor
Create a method on Fish named getInfo that takes no parameter and returns a string that includes the common_name, flavor, and record_weight for the fish. When called on $bass, getInfo might return “A Largemouth Bass is an Excellent flavoured fish. The world record weight is 22 pounds 5 ounces.”
<?php
class Fish {
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record){
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo(){
return "Fish Name: ". $this->common_name;
return "flavor: ". $this->flavor;
return "record: ". $this->record_weight;
}
}
$bass = new Fish("Largemouth Bass" ,"Excellent" ,"22 pounds 5 ounces");
?>
2 Answers
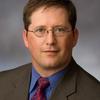
Ted Sumner
Courses Plus Student 17,967 PointsYour problem is that you did not follow the instructions on what to return. This code passed for me:
<?php
class Fish {
public $common_name;
public $flavor;
public $record_weight;
function __construct($name, $flavor, $record) {
$this->common_name = $name;
$this->flavor = $flavor;
$this->record_weight = $record;
}
public function getInfo() {
$answer = 'A' . $this->common_name . ' is an ' . $this->flavor . ' flavored fish. The world record weight is ' . $this->record_weight . '.';
return $answer;
}
}
$bass = new Fish('Largemouth Bass', 'Excellent', '22 pounds 5 ounces');
?>
The only difference that I see between our codes is the text of what getInfo returns.
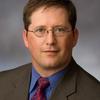
Ted Sumner
Courses Plus Student 17,967 PointsI am just now starting to really understand classes. Here is an overview from a site I came across that was really helpful:
OOP was designed to allow programmers to more easily model their programs upon real world scenarios - programmers literally define things (objects) in their world (program), set a few basic properties, then ask them to do things. Consider an object of type "dog" - there are many dogs in the world, but only one animal "dog". As such, we have a blueprint for dogs, from which all dogs are made. While dogs have different breeds that vary a great deal, at the end of the day they all have four legs, a wet nose, and a dislike of cats and squirrels.
So, we have our dog blueprint, from which we might create a Poodle breed, a Chihuahua breed, and an Alsatian breed. Each of these are also blueprints, but they are based upon the Dog blueprint. From our Poodle breed we can then create a Poodle, which we will call Poppy. Poppy is an actual dog, based upon the Poodle breed, and therefore also based upon the Dog blueprint. We can create other Poodles (or indeed Chihuahuas or Alsatians) simply by creating an instance of that breed.
As all dogs are able to bark, we can add a bark() function to our dog blueprint, which in turn means that the Poodle breed has a bark() function and therefore Poppy can bark() too. We can also define variables inside the dog blueprint such as $Name, $Age, and $Friendliness - again, this becomes available in the Poodle breed, which stems from the dog animal, and therefore also into Poppy. Each object of type Poodle would have its own set of variables - its own $Name, its own $Age, etc.
Because the breeds stem from the Dog blueprint, we can also add functions and variables to breeds individually without having them in the Dog blueprint. For example, Poodles come in three general sizes: standard, miniature, and toy. Last time I checked, you don't get toy Alsatians, and so putting a $Size variable into the Dog blueprint would just create a variable that is not used in one-third of the dogs.
If you're still with me, then you're on the way to fully understanding how object-oriented code works - there is a lot more to it, but we'll be getting there!
http://www.hackingwithphp.com/
When we use a class and its properties (variables) we have some powerful choices. From the example above, assume we had hundreds of dogs we were working with at the same time. The program could not keep track of what the property value was for any given dog since they all use the same properties. Thus, they made the $this variable so that there is a way for the program to distinguish between the properties for each instance.
Edward Palmer
3,313 PointsEdward Palmer
3,313 PointsIn the getInfo function, why do you have to give the properties as "this->common_name" and not just use "$name".
I don't really understand why we have gone to the trouble of assigning the properties earlier in the code only now to have to use the $this pseudo variable all over again.