Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial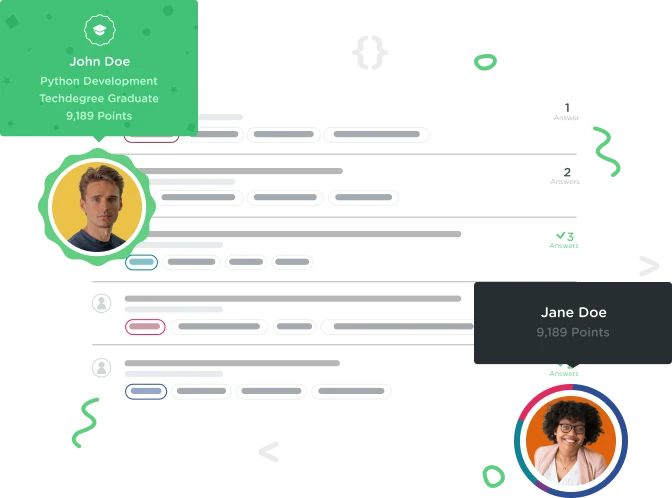

Nick Stoneman
10,093 PointsCreate a method on the contact object called fullName, use the programming from the printFullName function.
Stuck on this challenge question, and not clear about what is being asked for.
My code is below:
function printFullName() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
var contact = {
function fullName() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
}
4 Answers
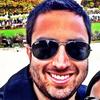
Pedro Marques
5,697 Pointsvar contact = {
fullName: function printFullName() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
}
Alternatively, you could just make the function anonymous as in "function()". You don't really need to call it "printFullName" or whatever :)
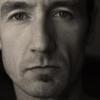
danheffernan
10,004 PointsTake a closer look at how you've created your object literal. The firstName and lastName properties aren't quite set up properly. Remember that key value pairs are expressed like so within an object literal: firstname: "Dan" with each property and value separated by a comma. Methods are setup much the same way within the object literal like so: printFullName: function() { do something }

Saqib Ishfaq
13,912 PointsSergey Podgornyy i don't think in this particular question, using ' this ' is the requirement! And for everyone else....... what's being asked here is to simply create a method called fullName on contact object like this>>>
var contact = {
fullName : function printFullName() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
}
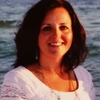
Robin Burton
2,481 Pointsvar contact = {
firstName: "Andrew",
lastName: "Chalkley",
fullName: function() {
console.log(firstName + " " + lastName);
}
}
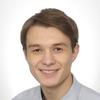
Sergey Podgornyy
20,660 PointsRobin, it will not work. You need access this variables with this
:
var contact = {
firstName: "Andrew",
lastName: "Chalkley",
fullName: function() {
console.log(this.firstName + " " + this.lastName);
}
}