Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial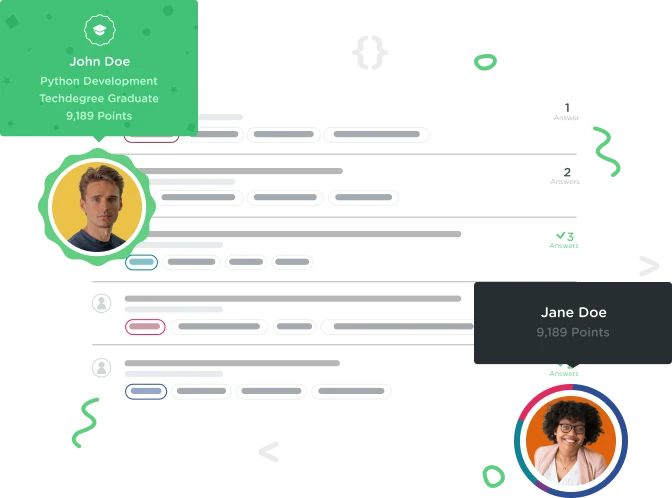

Khaleel Yusuf
15,208 PointsCreate a new Board subclass named TicTacToe.
Create a new Board subclass named TicTacToe. Have it automatically be a 3x3 board by automatically setting values in the init.
I don't know why this isn't working.
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self, width, height):
super().__init__(width=3, height=3)
4 Answers
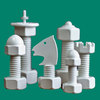
Steven Parker
229,785 PointsYou can't establish default values in a function call (the "super" line), but you could establish them in the function definition (the "def" line). As it is now, the constructor requires a width and height value even though it does not use them.
However, for this challenge, it's fine if the constructor for "TicTacToe" does not take dimension arguments at all and always uses 3x3 as the dimensions. Your "__init__
" override could take no arguments (except "self"), and you could just pass 3's in the "super" call.
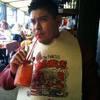
Paul Franco
28,480 Pointsclass TicTacToe(Board): def init(self): super().init(3, 3)
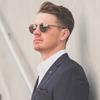
Cameron Sprague
11,272 PointsThis is how I solved this. If anyone has any questions about the code below please feel free to ask.
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self, width=3, height=3):
super().__init__(width, height)
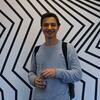
Danylo Onyshchenko
3,472 Pointsit is not working

Khaleel Yusuf
15,208 PointsWhat do you mean?
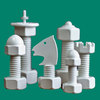
Steven Parker
229,785 PointsI expanded my answer a bit, hopefully it's more clear now.

greyskymedia
Courses Plus Student 3,948 Pointsclass Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self):
super().__init__(width=3, height=3)