Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial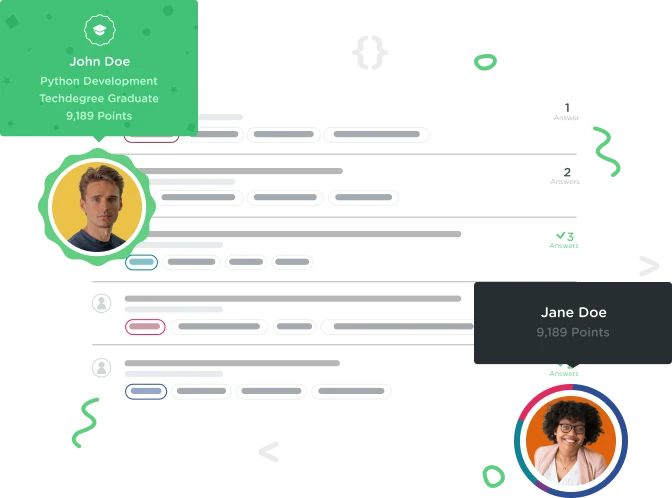

Dennis Fayard
7,567 PointsCreate a new function named max which accepts two numbers and I get msg 'max' function isn't returning a number.
Create a new function named max which accepts two numbers as arguments (you can name the arguments, whatever you would like). The function should return the larger of the two numbers.
HINT: You'll need to use a conditional statement to test the 2 parameters to see which is the larger of the two.
function max(num1,num2) { var num1 = prompt("Please enter first number"); var num2 = prompt("Please enter second number");
if (num1 > num2) { return num1; } else { return num2; } }
function max(num1,num2) {
var num1 = prompt("Please enter first number");
var num2 = prompt("Please enter second number");
if (num1 > num2) {
return num1;
}
else {
return num2;
}
}
4 Answers
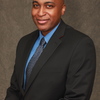
Bryan Knight
34,215 PointsHi Dennis,
I think you were over thinking this one. You have the answer but you need to remove the
var num1 = prompt("Please enter first number");
var num2 = prompt("Please enter second number");
What's happening is num1 and num2 are being passed into the function but those two lines immediately overwrites the values of num1 and num2 with the prompt value which is not needed. All you need is
function max(num1, num2){
if(num1 > num2){
return num1
}else{
return num2
}
}
Once you learn ternary operator you can do this in one line
function max(num1, num2){
return num1 > num2 ? num1 : num2;
}
FYI as a side note, prompt
returns a string. So best practice would be to parseInt()
the returned value of prompt
var num1 = parseInt(prompt("Please enter first number"));
var num2 = parseInt(prompt("Please enter second number"));
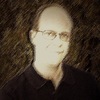
Jason Anders
Treehouse Moderator 145,858 PointsHey Dennis,
Your code is pretty much fine, except you are adding two lines that the challenge didn't ask for. If you delete the two var
lines you added, you'll be good to go.
function max(num1,num2) {
if (num1 > num2) {
return num1;
}
else {
return num2;
}
}
Keep Coding!
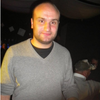
Vittorio Somaschini
33,371 PointsHello Dennis,
Quick solution here: the challenge does not required any input, just set up the function as you did but get rid of the inputs and the compiler will take care of the input to test your code.
Vitto

Anthony Branze
4,887 PointsMy solution ddidnt use an else.
max(a,b) {
if (a<b){
return b;
}
}