Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial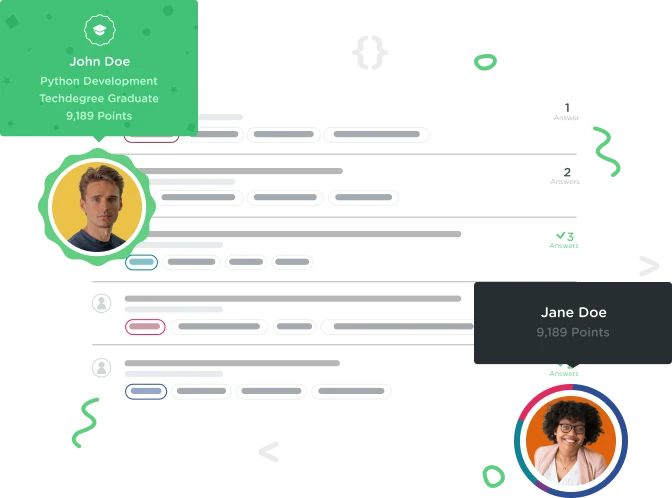

Ryan Roy
Courses Plus Student 3,586 PointsCreate a new function named max() which accepts two numbers as arguments. The function should return the larger of the 2
Having trouble with this one the other comments I found on the site we're not helpful to me.
function max(a,b){ var first = a; var second = b; if first > second { return("first is greater"); } else { return("second is greater"); } max(4,5)
function max(a,b){
var first = a;
var second = b;
if first > second {
return("first is greater");
} else {
return("second is greater");
}
max(4,5)
6 Answers
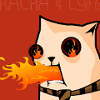
Brian Romine
9,518 PointsI can see a couple issues in your code. The first is a syntax error.
foo = "bar";
if ( foo == "bar" )
{
foo = "something";
}
is the general syntax for an If statement. You will need to add (first > second) for your condition test.
Second, a style issue. Why define the var first and the var second? Would it work to just test if (a > b) ?
Third, I see a minor logic issue. What about an edge case where a === 3 and b === 3? You'll get a result that the second is greater, maybe add an "else if" clause? (that might be beyond the scope of your challenge)
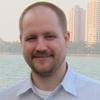
Ryan Field
Courses Plus Student 21,242 PointsIn addition to what Brian has said, you are missing a final closing curly brace (}
) for your function, which will cause an error.
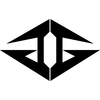
Julian Gutierrez
19,201 PointsLike mentioned above you need a closing bracket for your function but the challenge also need you to return the largest of the two numbers not a string. You would need to return a or return b.

Ryan Roy
Courses Plus Student 3,586 PointsI found this was the solution that worked for me. Yes I was missing the extra bracket and returning a string. I may have been over thinking the question. Thanks for all your help guys. I really appreciate the support.
function max(num1, num2) { if (num1 > num2) { return num1; } else{ return num2; } }
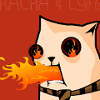
Brian Romine
9,518 PointsGood job!
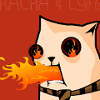
Brian Romine
9,518 Pointsfunction max(a,b) {
if (a > b)
{
return("first is greater");
}
else
{
return("second is greater");
}
max(4,5);
Still two issues. Look carefully at your brackets. You need a closing bracket for every opening bracket. Also, like Julian said, you should be returning the larger number.

Ryan Roy
Courses Plus Student 3,586 PointsThanks guys, This is where I am now based on the comments. It's still not passing however.
Here is the question again so you don't have to scroll up: Create a new function named max() which accepts two numbers as arguments. The function should return the larger of the two numbers. You'll need to use a conditional statement to test the 2 numbers to see which is the larger of the two.
function max(a,b){ if (a > b) { return("first is greater"); } else { return("second is greater"); } max(4,5);