Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial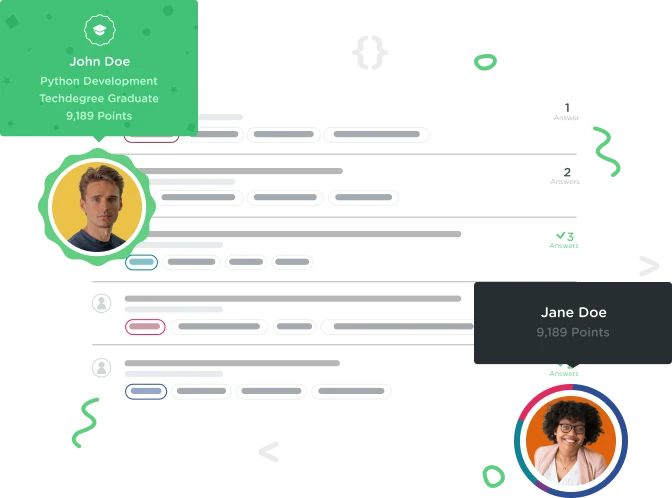

Susannah Klanecek
5,825 PointsCreate a new int instance from __new__
Now override new. Create a new int instance from whatever is passed in as arguments and keyword arguments. Return that instance. You should remove the pass.
class Double(int):
def __new__(*args, **kwargs):
4 Answers
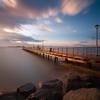
Qasa Lee
18,916 Pointsclass Double(int):
def __new__(*args,**kwargs):
return int.__new__(*args,**kwargs)
Here is what I've done to pass that challenge. Perhaps it's helpful to other visitors.
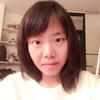
Xiang Liu
24,339 PointsThanks for your code. It helps me to learn.
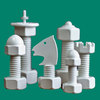
Steven Parker
231,623 PointsYou're good so far, but you haven't finished this task yet. You still need the body of the method where you call the __new__
of the base class and then return what it creates.
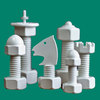
Steven Parker
231,623 PointsI'd appreciate feedback from whoever down-voted this answer to help me improve.
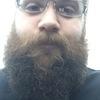
Jebadeiah Jones
10,429 PointsSomething tells me it was down-voted because it didn't explicitly give out the answer.
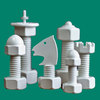
Steven Parker
231,623 PointsYou may be right, but that's not a good use of downvoting. I think most people have a better learning experience when they resolve an issue themselves with assistance rather than having it resolved for them. The fact that in the last year it got enough up-votes to make it no longer possible to tell it was ever downvoted ir probably a good indication that many other students agree.
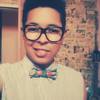
Tatiane Lima
6,738 PointsI Wrote this code:
class Double(int):
def __new__(self, *args, **kwargs):
try:
item = int.__new__(self, *args, **kwargs)
except:
item = self.__new__(self, *args, **kwargs)
return item
It was showing me an error message, then i got that i need to treat the error. Then i storaged the atribute passing by int method in a variable and check if it is an int type, if it is not, i do the same but i do it using the "self" notation.
I'm not sure if it is the right way to do it, but it worked.
(sorry about my english)
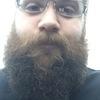
Jebadeiah Jones
10,429 PointsFirst, there's no need to apologize for your English. It reads well.
From what I can tell, your code is redundant. I'm guessing you started with the 'try' block and the error that popped was saying there was no 'except' case. Putting in the except case removed the error, but didn't fix the problem. It actually never gets read.
I may be wrong, but what I see is the code 'trying' to assign the variable 'item' the value of:
int.new(self, *args, **kwargs)
This will succeed every time, as you're initializing a variable and assigning it a value in the same phrase. Because your 'try' is programmed to succeed everytime, your code will never touch the 'except' case, though it's required for every 'try' case.
TLDR: Instead of trying to assign a variable called 'item' the value of ""int.new(self, *args, **kwargs)"", simply return that value.

Shavez Memon
Python Development Techdegree Student 2,452 PointsThis worked for me:
class Double(int):
def __new__(self, x):
return int.__new__(self, x)
Susannah Klanecek
5,825 PointsSusannah Klanecek
5,825 Pointsthe def new(*args, **kwargs): is my code, it could be wrong!