Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial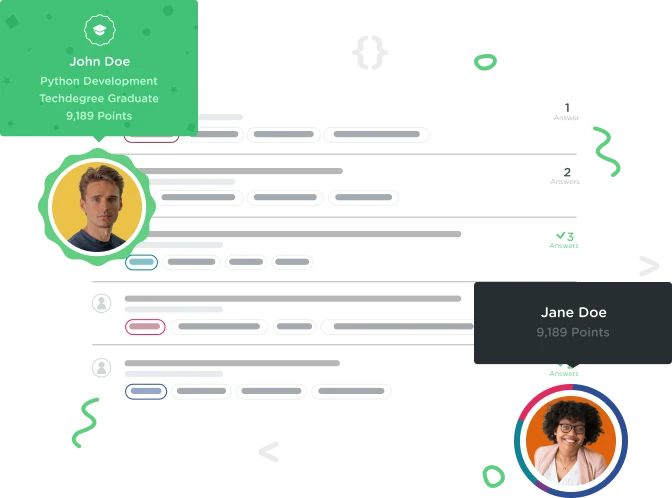

Naval Kulkarni
13,782 PointsCreate a variable named matchingBirds and assign it a LINQ query on the birds variable that have the same Color property
Create a variable named matchingBirds and assign it a LINQ query on the birds variable that have the same Color property as the mysteryBird object. In the query, return an anonymous type with a property named BirdName and assign to it the Name property of the birds.
var birds = new[]
{
new { Name = "Pelican", Color = "White" },
new { Name = "Swan", Color = "White" },
new { Name = "Crow", Color = "Black" }
};
var mysteryBird = new {Name="MysteryBird", Color = "White", Sightings =3 };
var matchingBirds = from b in birds where mysteryBird.Color == "White" select new { BirdName = b.Name,BirdColor=b.Color};
1 Answer
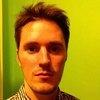
Brendan Whiting
Front End Web Development Techdegree Graduate 84,736 PointsThe problem is your where
condition: where mysteryBird.Color == "White"
. The mysteryBird color is white, so this boolean expression will always be true, which is why we end up with all 3 birds in our result, rather than filtering it down to just 2.
What you want is to check if each bird, b
, in the loop has the same color as the mysteryBird: mysteryBird.Color == b.Color
var birds = new[]
{
new { Name = "Pelican", Color = "White" },
new { Name = "Swan", Color = "White" },
new { Name = "Crow", Color = "Black" }
};
var mysteryBird = new {Name="MysteryBird", Color = "White", Sightings =3 };
var matchingBirds = from b in birds where mysteryBird.Color == b.Color select new { BirdName = b.Name,BirdColor=b.Color};