Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial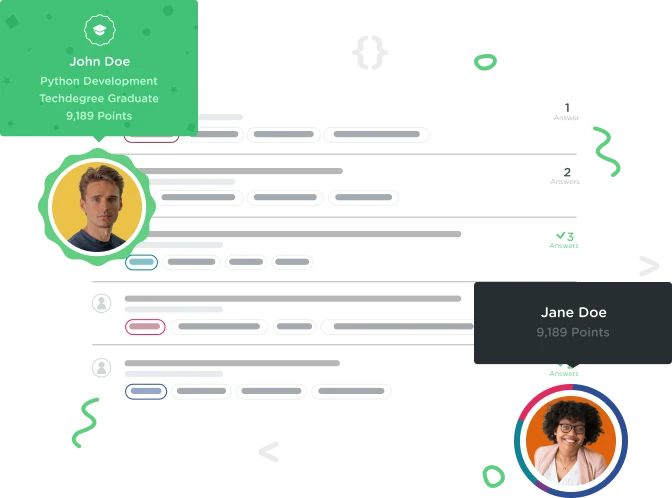

Dmitry Dell
6,940 PointsCreate Function. What's wrong with the code?
I created two versions of code, each work when I test in a separate workstation. But none lets me pass through the "Check Work". What might be wrong with it? TASK: Create a function named square. It should define a single parameter named number. In the body of the function return the square of the value passed in. (A square is the value multiplied by itself. eg: The square of 5 is 25. 25 = 5 x 5)
Version 1:
def square(number): return float(number) * float(number) input_number = input("What is the number? ") print(square(input_number))
Version 2:
def square(number): number_2 = number * number return number_2
input_number = float(input("What is the number? ")) answer = square(input_number) print('the square of the value is {}'.format(answer))
def square(number):
number_2 = number * number
return number_2
input_number = float(input("What is the number? "))
answer = square(input_number)
print('the square of the value is {}'.format(answer))
3 Answers
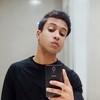
Jesse Davidson
647 PointsHi Dmitry
The return function will only return a value of your function. Basically the output or the end result to say.
Example your code:
Leave out float as this as this is data type that returns decimal points. The objective is to square a number.
There are two ways of doing this:
1st option: This would be returning the value of number * number. So when passing the argument through number it will return it's value.
def square (number): return number * number
or
2nd option: All what was done was store the the number * number calculation in a variable and then return the value of this variable.
def square (number): number_squared = number * number return number * number
This way I'm defining my function with the parameter named (number) and returning the value of |number*number|
Example of a written out code:
Defining my function
def square(number): return number * number
I used an integer data type so that it's whole number when the user inputs there value.
the_number = int(input("What is your number you would like square? "))
I'm passing the arguement called the_number into my function
persons_number = square(the_number)
I'm printing out the variable that contains my function.
print("The number squared is {}. ".format(persons_number))
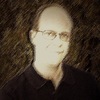
Jason Anders
Treehouse Moderator 145,860 PointsHi Dmitry,
You've got the first task correct, although you could shorten the code a bit. Remember to always try and code D.R.Y. For the first task, you just need to return the value, there is no need to create a new variable. All that is needed is:
def square(numb):
return numb * numb
As for the second task, I don't see any of the code asked for by the instructions. Those three lines of code you have, whiile they look like they are syntactically correct, it is not what the instructions are asking for. For the second task you just need to create a variable and store the return of the function called with 3
.
variable_name = function(parameter)
You'll need to delete those three lines of code you have and replace it with just the single line of code the challenge is expecting. Remember for future challenges that they are very picky and the instructions must be followed exactly or the task will fail (even with working code).
Hope that helps.
Nice work, though! :)

Brad Thurlow
7,809 Pointsdef square (number):
return number ** 2
'''