Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial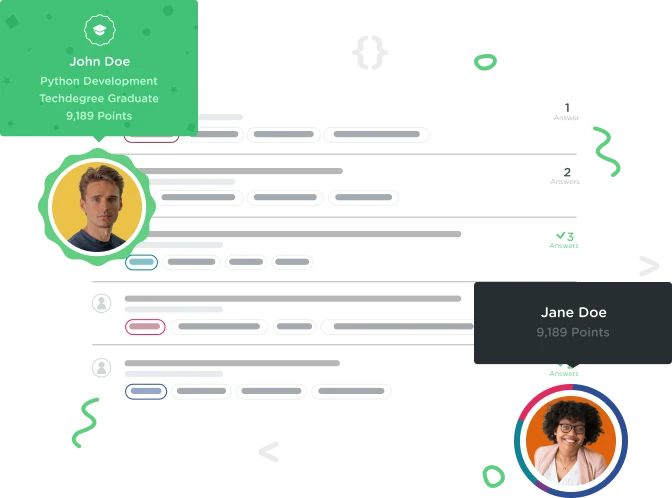

kevinthecoder
10,791 PointsCreate Random Website Background Color on Mouseclick. Need some help/direction please.
Hello! I'm doing my own mini-project where I'm trying to create a random background color to appear on the entire blank webpage EVERY time the user clicks their mouse button anywhere on the blank page. It should have nothing to do with clicking on links. I am using workspaces.
I thought about a mouse click event but that may be too complicated for me as I'm a newbie. Yes, I have taken Javascript Basics and jQuery courses. I have thought about using the pseudo item :active but that only gets me so far.
Here is what I did for the initial setup with a simple HTML/CSS file just for me to start thinking it through. I can get a blank webpage to work with just one color only by doing this with CSS:
html:active {
background: green;
}
Again, that works with only color only and the color goes back to a blank white page when the user releases the mouse button.
Here is my sample HTML code. I am keeping it very simple:
<!DOCTYPE html>
<html>
<head>
<title>Change colors on a Webpage</title>
<link rel="stylesheet" href="css/style.css" type="text/css" charset="utf-8">
</head>
<body>
<h1>Click anywhere on the page to make it change color</h1>
</body>
</html>
I would really like to use Math.random() and I think it could work with RGBA with something like this in Javascript: var randomColor = 'rgb(' + (Math.floor(Math.random() * 256)) + ',' + (Math.floor(Math.random() * 256)) + ',' + (Math.floor(Math.random() * 256)) + ')';
However, I'm a newbie and I don't know how to return it or tie it into CSS or by using some other method. I know that I probably need to tie this in via 'background' in some way. I've done various searches on the internet and I can't find anything specific so that I can understand it. What I need is some guidance on how to proceed to the next step.
By the way, this is EXACTLY why I'm considering bootcamps and have done careful research on several of them already. I need to learn 'how to learn'. I need a 'push' . I need an explanation. I need to be given a 'direction' or advice by an instructor. I don't need the instructor to give me the full answer and solve my problem for me; I just need them to guide me to the next step/process so that the brain starts working again. I am 'stuck' and I need to know how to proceed to take it to the next level/step. Yes, I am going to pair programming sessions but it is only every other week in my city and it is only for two hours, which stinks; I need to be challenged EIGHT HOURS A DAY, not two hours every two weeks. I am an information sponge and I need constant challenges and being bombarded by motivated teachers/instructors. So far, I have only done 7 challenges on github.
I really hope this makes sense. Sorry for the long explanation but I just wanted to state where I am coming from. Thanks for reading.
Can anyone help or advise me? Thanks!
5 Answers

kevinthecoder
10,791 PointsRepost of HTML code as it's now showing everything:
<html> <head> <title>Change colors on a Webpage</title> <link rel="stylesheet" href="css/style.css" type="text/css" charset="utf-8"> </head> <body> <h1>Click anywhere on the page to make it change color</h1> </body> </html>
I can't get all the code to post. Argh. Sorry!
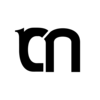
Colin Marshall
32,861 PointsSomething like this?:

kevinthecoder
10,791 PointsYES!!
However, a couple questions are in order. So the .css line at the end has 'background-color'. Couldn't it be done with just 'background'? or must we use background-color?
Why are they using .preventDefault?
OK, so that uses jQuery since it's using the $; got it. So jQuery refers to the body in HTML and CSS; interesting. So it appears that jQuery is passing in two arguments (I hope I said that right); the first argument is the type of CSS pseudo selector/type (background-color) and the second argument is the random RGB color. I'll have to look up jQuery and see their explanation of arguments that you can bring in plus how many arguments total are allowed in (I am guessing that it's just one or two only and no more).
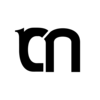
Colin Marshall
32,861 Pointsbackground-color and background will both work.
I used preventDefault to prevent the default action that happens when you click on the document. It will probably work fine without it in this situation, but it is necessary to have in most click events. It's like saying "Do this, instead of what you normally do."
You are correct that I used jQuery. It is not necessary, but I am more efficient with it. The .css()
method will accept 1 or 2 arguments. If you only pass one argument, 'background-color' for example, then it will return the value for 'background-color' of the element you are calling it on. If you were to pass a 2nd argument through it, it will set the value for that CSS property, as I have done in my example.

kevinthecoder
10,791 PointsColin,
Thanks for your explanation! I will try both background types to see what I get. I also looked up the W3schools explanation of the jQuery .css method; I mostly understood it; my problem is that I wouldn't have known to have picked it in the FIRST place (as expected, since I'm a newbie). I am hoping that things get easier as I progress, as I build stuff and as I proceed through bootcamp hopefully.
A couple observations:
Instead of the preventDefault, could we have put in 'return false' at the same line entry (I plan to try this shortly)? Or is this not relevant in this case?
Also, I like the combination of math.floor with math.random as it guarantees that we CANNOT get a (bad) value of 256 ever. The javascript track also covered both functions.
Again, thanks for breaking it down for me. :) I tend to like the details.