Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial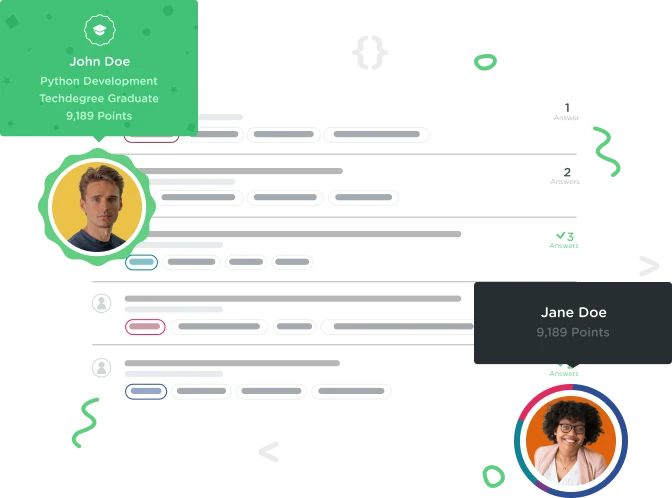

Sharon Kearney
8,662 Pointscreate watcher
code challenge help
$scope.$watch ('user.id' function (getUserData) { if (newId) { api.saveId ($scope.userId); }
});
})
angular.module('myApp', [])
.controller('myController', function ($scope, $http) {
$scope.user = {name: 'Alex', id: 123};
getUserData = function (id) {
console.log('Getting user with ID: ' + id);
}
$scope.$watch('user.id' function (getUserData) {
if (newId) {
api.saveId ($scope.userId);
};
});
});
<!DOCTYPE html>
<html ng-app="myApp">
<head>
<title>Angular.js</title>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.2/angular.min.js"></script>
<script src="app.js"></script>
</head>
<body ng-controller="myController">
<input type="text" ng-model="user.id" />
</body>
</html>
1 Answer

Andrew Kiernan
26,892 PointsHi Sharon!
The benefit of the $scope.$watch
is that you don't need to check the property to see if it changed, the callback function you pass into $scope.$watch will only be executed when the property you are watching changes. It will also pass the changed property into the callback. With this in mind, you can just pass the function you want to execute into the 2nd parameter of the $watch method.
$scope.$watch('user.id', getUserData);
Hope that helps! Let me know if you have any questions.
-Andrew