Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial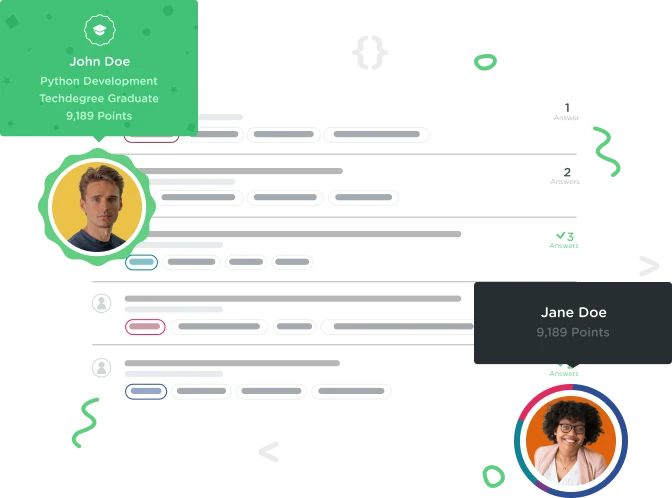
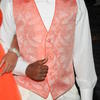
Rashii Henry
16,433 PointsCreating a Data Model To Encapsulate Values That Will Become The textTitle.text Attributes Of A Custom Table View Cell?
This question may seem a little weird but let me simplify and give you a visual.
You have a UITableView in storyboard, along with the custom UITableView class in the project navigator. Lets say the the title of this custom class is CTableViewController.
You want to customize your tableViewCells accordingly, Therefore you have to create another custom class. This being a subclass of UITableViewCell. following the same naming model, CTableViewCell.
You've added a UILabel to your CTableViewCell header and connected it via storyboard:
@property (nonatomic, strong) IBOutlet UILabel *titleLabel;
Here's the viewDidLoadMethod in CTableView
- (void)viewDidLoad
{
[super viewDidLoad];
_moodsArray = @[@"Mad",
@"Dazed",
@"Happy",
@"Strange",
@"Intense"];
}
Now in CTableView cellForRowAtIndePath: depending on the index of the array count you want to show that objects index as the title, i've created this for loop with nested if statements, that works.
- (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//make the custom cell class create its own cell and use the reuse idenifier from storyboard.
moodTableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"Cell" forIndexPath:indexPath];
//this also works to set the text to the right indexPath
// int row = [indexPath row];
// cell.titleLabel.text = _Moods[row];
//go through the count of the array and according to the indexPath set its titleLabel.
for (int i = 0; i < [_moodsArray count]; i++)
{
static int defaultTextSize = 24;
if (indexPath.row == 0)
{
cell.titleLabel.text = [_moodsArray objectAtIndex:0];
cell.titleLabel.font = [UIFont fontWithName:@"Heiti TC" size:defaultTextSize];
cell.backgroundColor = [UIColor blackColor];
// cell.detailTextLabel.text = @"The Moods Here";
}
else if (indexPath.row == 1)
{
cell.titleLabel.text = [_moodsArray objectAtIndex:1];
cell.titleLabel.font = [UIFont fontWithName:@"Heiti TC" size:defaultTextSize];
// cell.detailTextLabel.text
}
else if (indexPath.row == 2)
{
cell.titleLabel.text = [_moodsArray objectAtIndex:2];
cell.titleLabel.font = [UIFont fontWithName:@"Heiti TC" size:defaultTextSize];
}
else if (indexPath.row == 3)
{
cell.titleLabel.text = [_moodsArray objectAtIndex:3];
cell.titleLabel.font = [UIFont fontWithName:@"Heiti TC" size:defaultTextSize];
}
else if (indexPath.row == 4)
{
cell.titleLabel.text = [_moodsArray objectAtIndex:4];
cell.titleLabel.font = [UIFont fontWithName:@"Heiti TC" size:defaultTextSize];
}
/*
//mood being a Mood object and then mainMoods being a property of The Mood object that has an NSArray holding the main moods
cell.titleLabel.text = [self.mood.mainMoods objectAtIndex:4];
*/
}
return cell;
}
All of this works flawlessly, But after following Pasan Premaratne in creating a Data Model, i did the same by creating a subclass NSObject to make sure my code is extremely efficient.
Therefore, I created a subclass of NSObject named Mood, that contains the array of moods.
I created the init method inside of this subclass and initialized the array within the same scope. then went back to CTableView and changed every line that said '_moodsArray' to 'self.mood.mainMoods'
mainMoods being the array property of the Mood object.
When i compile my code, there are no compiler errors or runtime Errors, the titles just don't appear. Would it make a difference if i used a NSManagedObject subclass?
Any suggestions? Ben Jakuben Amit Bijlani Ash Furrow Stone Preston
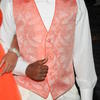
Rashii Henry
16,433 Pointswow, that was the solution right there.
I overlooked alloc and initializing it in the viewDidLoad of CTableView simply because i thought the CTableView was grabbing the array from the Mood object(which it is). For some reason i thought if i just initialized the NSArray in the object(Mood) itself, it would somehow override the CTableView's viewDidLoad method. I tried to take a shortcut.
Thanks.
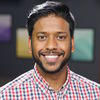
Pasan Premaratne
Treehouse TeacherWithout the alloc/init the property was nil and messaging nil in Objective-C silently fails which is why you weren't seeing any errors :)
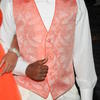
Rashii Henry
16,433 PointsThanks Pasan,
i like the way you teach your courses. Do you think you guys could release a course on using instruments to test performance for complex applications.
I recently did an interview with (http://ingeniousmed.com/) they recruited me because of my profile, i nailed the interview.
but since i had no experience with instruments(didn't know it was hiding in xcode) they told me i had great skills but they picked someone else for a job.
i have faith in you guys, otherwise I wouldn't have landed the interview. It was a great experience.
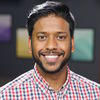
Pasan Premaratne
Treehouse TeacherMost certainly can!
Pasan Premaratne
Treehouse TeacherPasan Premaratne
Treehouse TeacherI'd need to see your entire project to really debug. Off the top of my head, did you alloc/init the mood property?