Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial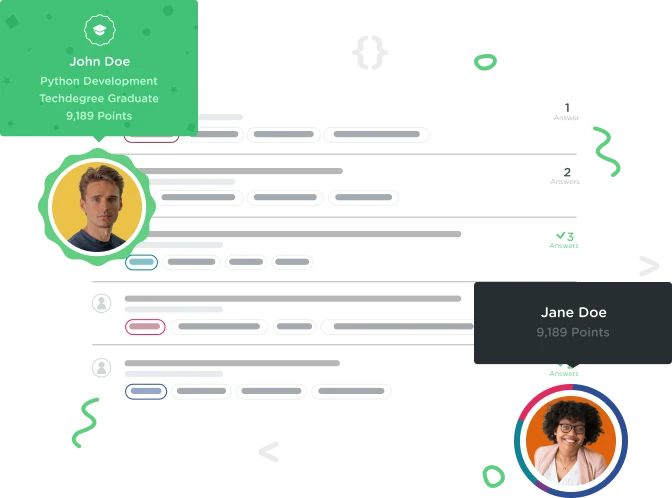

Michael Aronian
Python Web Development Techdegree Student 5,453 PointsCreating a function to return the first 4 items and last 4 items of a list
I can't seem to figure out why I am not getting the right items in the list.
def first_4(items):
return items[0:4]
def first_and_last_4(items):
return(items[0:4] + items[-6:-2:1])
4 Answers
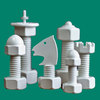
Steven Parker
231,269 PointsThe slice expression "items[-6:-2]
" would give you the sixth-from-last up to (but not including) the second-from-last.
But I think you intended to get the last 4 ("fourth-from-last to the end").
I'll bet you can get it now without an explicit code spoiler.
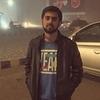
zainsra7
3,237 PointsHi,
Solution:
def first_4(items):
return items[0:4]
def first_and_last_4(items):
return(items[0:4] + items[-4:])
See that they're asking for last 4 items in the list, you've already done the first 4 correctly [0:4],
but for last 4 items , we need to start from the end of list so a negative number , now the thing is that item list could be of any length e.g 5, 500 , 5000 or 5 million items
and we need last 4 , so during slicing we give starting and ending end for a list as in [0:4] we're saying to return elements from 0 to 3 , 0-1-2-3 (total 4 items).
[-4:] => it will return last 4 items of any list. How ? Let's see
start from end of list, [-4] makes us go 4 steps backward from the list and at this point we're standing at the 4th item from the last of list. For the ending range we didn't specify any range as we want all the items from [-4] location to the end of list , so we left that part out.
I hope it answers your question, Happy Coding :)
- Zain

Michael Aronian
Python Web Development Techdegree Student 5,453 PointsThank you, I figure out what I was doing wrong right after I sent this in. But I am having trouble figuring out the last question. I was wondering if you could answer the reverse even index part of this.
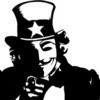
behar
10,799 PointsHey Michael! Yes the wording of this challenge is very vague, and i would be tempted to do the same as you, however, it actually wants you to return the list from index -4 and ahead. Your returning from -1 and going back to -4. Instead do it like this:
def first_and_last_4(items):
return items[0:4] + items[-4:]
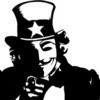
behar
10,799 PointsHey again Michael! The correct solution for the next challenge is:
def odds(itr):
return itr[1::2]
so were returning every index starting at index 1, thru to wherever the list end, and where going thru every other item, so it will go itr[1] - itr[3] - itr[5] etc.. Hope this helps!
Steven Parker
231,269 PointsSteven Parker
231,269 PointsOn the very last task, there's two ways to approach it. One would be to compute the starting position based on the size of the list, and the other would be to use one slice to get all the even-indexed values and then another slice to reverse the order.