Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial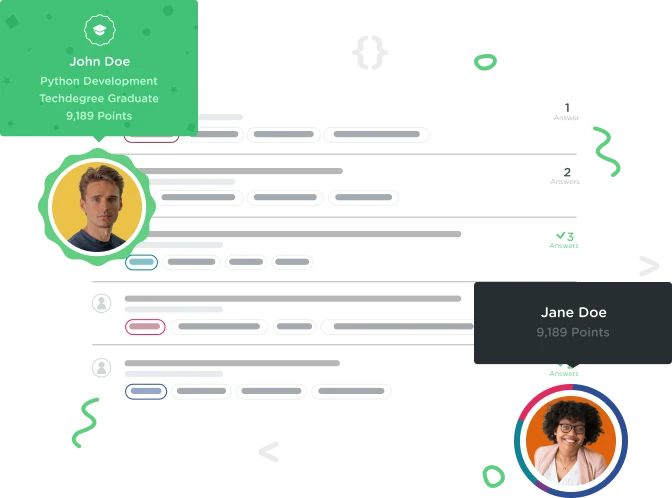

Jun Kakeno
iOS Development with Swift Techdegree Graduate 24,842 PointsCreating a generic type 5/5
I've tried this on the playground and it works. I'm not sure why it doesn't pass the challenge. Please help.
struct Queue<Element>{
var array=[Element]()
var isEmpty: Bool {
if array.count == 0 {
return true
} else { return false }
}
var count:Int{
return array.count
}
mutating func enqueue(_ element: Element) {
array.append(element)
}
mutating func dequeue()->Element?{
if array.count != 0{
var first = array[0]
array.remove(at: first as! Int)
return first
}
return nil
}
}
2 Answers
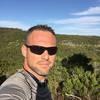
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsHi Jun, here are some things to change:
- Remember that we already created the computed property 'isEmpty' so use that instead in 'dequeue'.
- Also it states that 'first' should be an optional. So technically you only need one return since it will either be nil or not, no need for a nil return.
- You can use array[0] or array.first.
- You can use array.remove(at: 0) or array.removeFirst()
struct Queue<Element>{
var array: [Element] = []
var isEmpty: Bool {
if array.count == 0 {
return true
} else { return false }
}
var count:Int {
return array.count
}
mutating func enqueue(_ element: Element) {
array.append(element)
}
mutating func dequeue() -> Element? {
var first: Element?
if isEmpty == false {
first = array.first
array.removeFirst()
}
return first
}
}
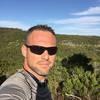
Brandon Mahoney
iOS Development with Swift Techdegree Graduate 30,149 PointsYour welcome and if it worked please remember to upvote and mark me best answer.