Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial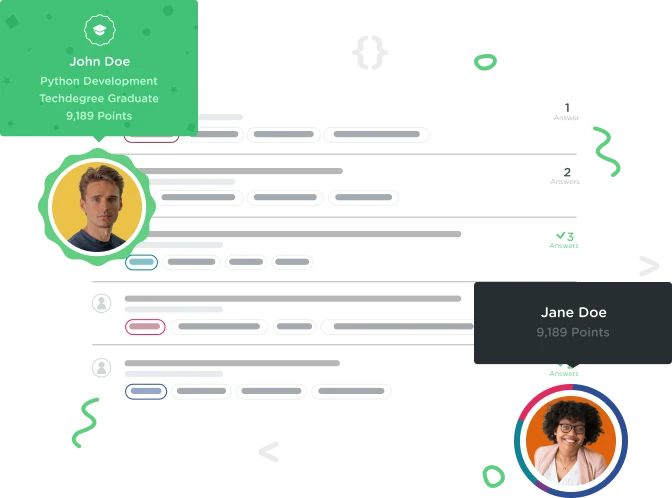

Bob Boursaw
2,047 PointsCreating a new object and setting the value via the constructor.
The LandingActivity interacts with the Spaceship model object we created. Start by setting mSpaceship to a new Spaceship object in the onCreate() method. Use the custom constructor that takes a String parameter, and pass in "FIREFLY" as the parameter.
I am not sure if this is me or the way the question is written, but I am missing something. The question for this challenge states the following.
The LandingActivity interacts with the Spaceship model object we created. Start by setting mSpaceship to a new Spaceship object in the onCreate() method. Use the custom constructor that takes a String parameter, and pass in "FIREFLY" as the parameter.
So the first part I thought would be very straight forward. We have the member variable mSpaceship already defined in the code, so I put the following in the OnCreate method.
mSpaceship = new Spaceship();
However, doing this results in an error stating "Make sure you set the type for mSpaceship.
So I changed it to this.
Spaceship mSpaceship = new Spaceship();
Then I get an error that says, "Looks like you aren't setting mSpaceship in the onCreate() method, which is really interesting since I didn't move my code, I just added to it.
I realize the second part of this has not been done yet, and that is a bit confusing to me as well.
The instructions say, "Use the custom constructor that takes a String parameter, and pass in "FIREFLY" as the parameter."
The way that's worded, it sounds like the constructor should be there, but clearly it is not. I attempted to add a new constructor but the error I am receiving didn't change.
Any help here would be greatly appreciated.
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
public class LandingActivity extends Activity {
public Button mThrustButton;
public TextView mTypeLabel;
public EditText mPassengersField;
public Spaceship mSpaceship;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_landing);
mThrustButton = (Button)findViewById(R.id.thrustButton);
mTypeLabel = (TextView)findViewById(R.id.typeTextView);
mPassengersField = (EditText)findViewById(R.id.passengersEditText);
// Add your code here!
Spaceship mSpaceship = new Spaceship();
}
}
public class Spaceship {
private String mType;
private int mNumPassengers = 0;
public String getType() {
return mType;
}
public void setType(String type) {
mType = type;
}
public int getNumPassengers() {
return mNumPassengers;
}
public void setNumPassengers(int numPassengers) {
mNumPassengers = numPassengers;
}
public Spaceship() {
mType = "SHUTTLE";
}
public Spaceship(String type) {
mType = type;
}
}
2 Answers

Bob Boursaw
2,047 PointsThe issue turned out to be something jacked up in cache.
Shut down Chrome, reset all cache and launched in incognito mode.
The original code I had tried then worked without issue.
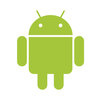
Jeremy Johnson
1,428 PointsNo, it's not right. i have the same problem. It turns out to be one line. mSpaceship = new Spaceship("FIREFLY");
Bob Boursaw
2,047 PointsBob Boursaw
2,047 PointsHi Jeremy,
I think the only thing that you are missing is to put firefly with" inside of your parentheses and that should do it. Then you could move onto the next part.