Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial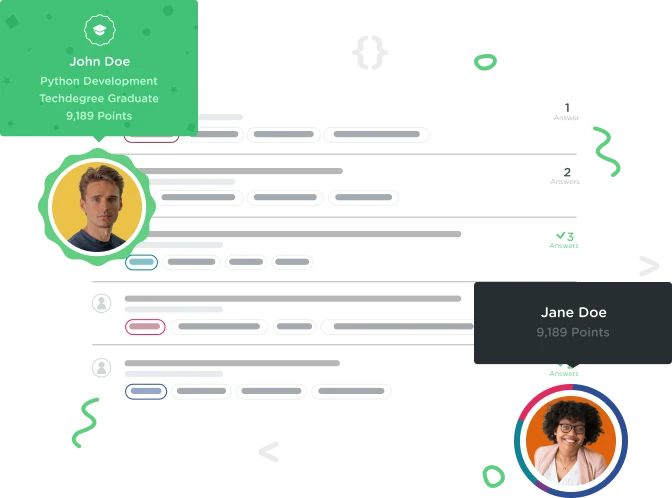

Bryan Noll
9,790 PointsCreating a Password Confirmation Form - function if username is present?
At the end of the video, Andrew talks about adding a function if there is a username present so that the form can be submitted. That is, in the project we disabled the submit button if the passwords don't match or they're 8 characters or less.
I've tried my hand at adding a function to check if a username is present:
function isUsernamePresent() {
return $username.val().length > 0;
}
But the submit button remains disabled even when a username is present. Does anybody have any tips?
Here's all of what I've got:
//Problem: Hints are shown even when form is valid
//Solution: Hide and show them at appropriate times
var $username = $("#username");
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
//Hide hints
$("form span").hide();
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function isUsernamePresent() {
return $username.val().length > 0;
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching() && isUsernamePresent();
}
function passwordEvent() {
//Find out if password is valid
if(isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//Else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if(arePasswordsMatching()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//Else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$("#submit").prop("disabled", !canSubmit());
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
//When event happens on confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent);
enableSubmitEvent();
2 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Bryan,
What I noticed was that the submit button would be enabled if you entered the username first and then did the passwords but if you did the username last then the button would remain disabled.
As you discovered, what you were missing is that the enableSubmitEvent
function wasn't being called in response to entering input in the username field.
The only way the submit button can be enabled is by calling enableSubmitEvent
and if canSubmit
is true then the button will be enabled.
The problem was that this function wasn't being called as you were filling out the username.
So if you enter username last then this function wouldn't get called anymore and the submit button couldn't be enabled.

Bryan Noll
9,790 PointsOh wow, I now see that the code challenge addresses my question. Awesome.

Bryan Noll
9,790 PointsAnd now that I've completed the code challenge and looked over the code, I see that I was missing this:
$username.focus(usernameEvent).keyup(usernameEvent).keyup(enableSubmitEvent);
And to make sure that I'm just generally understanding how JavaScript/jQuery works, I was missing the part that actually runs the variables and functions defined earlier in the code, right?
Any clarification is appreciated. I'm just trying to zoom way out and understand simply what is happening. To use the English language as an analogy, it's easy to get focused on the finer details of how to spell words and where to place punctuation, and not really understand sentence structure of nouns, verb, adjective, etc.
Bryan Noll
9,790 PointsBryan Noll
9,790 PointsThank you for the explanation, Jason. This really helped to clarify how it works.