Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial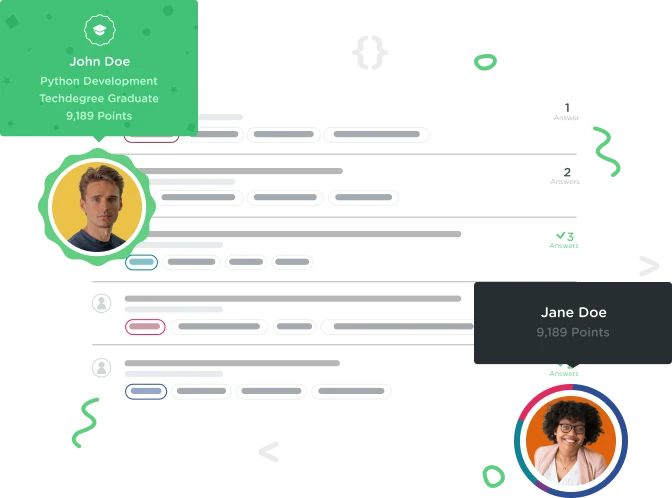
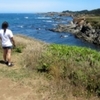
Nancy Melucci
Courses Plus Student 35,157 PointsCreating a recursive timer in the DOM with JavaScript
I am trying to make a JS function that will run a timer (like a clock) from 1 to 12 and then reset it using setInterval. I can't figure out how to make it run. I can toggle from AM to PM using setInterval, and change an image. But not this. Does anyone have a hint? My code is posted below. Just to be clear, the document text needs to change from sequentially to 12, and then reset to 1 and start again. This is why I figure it needs to be recursive...
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title>Untitled Document</title>
<head>
<script type="text/javascript">
function updateTime () {
var t = document.getElementbyId("output").value;
t = 1;
if t < 12;
setInterval (t++, 200);
else if (t === 12) {
t = 1;
updateTime();
}
}
updateTime();
</script>
</head>
<body>
<textarea id="output">1</textarea>
2 Answers
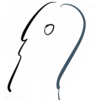
akak
29,445 PointsSolution based on your code:
function updateTime() {
var time = 1;
setInterval(function() {
document.querySelector("#output").value = time;
time++;
if (time > 12) {
time = 1;
}
}, 200);
}
updateTime();
solution I would go for so you can easily start, stop and customize the timer:
var timer = {
currentTime: 1,
startTime: 1,
maxTime: 12,
interval: 200,
target: "#output",
updateTime: function() {
document.querySelector(this.target).value = this.currentTime;
this.currentTime++;
if (this.currentTime > this.maxTime) {
this.currentTime = this.startTime;
}
},
start: function() {
this.currentInterval = setInterval(this.updateTime.bind(this), this.interval);
},
stop: function() {
clearInterval(this.currentInterval);
}
};
timer.start();
timer.stop();
Cheers!
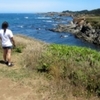
Nancy Melucci
Courses Plus Student 35,157 PointsThank you so much.