Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial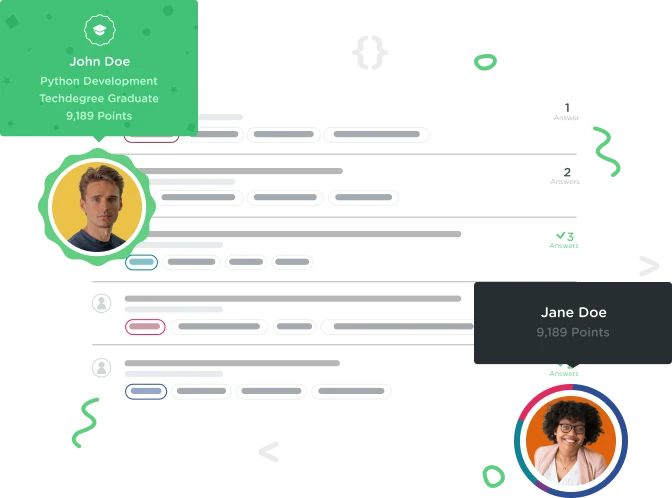
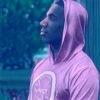
Marcus Alleyne
11,469 PointsCreating a setter and it is not working. Not sure where I am going wrong
error message says my setter method is set to the wrong property? I think my if statements are ok, not sure what needs to change.
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
set major(major) {
this._major = major;
if (this.credits == 'Senior' || 'Junior') {
return this._major;
} else {
return 'None';
}
}
}
var student = new Student(3.9, 60);
1 Answer

Dylan Irvin
12,577 PointsHi Marcus!
The if statement in the setter function asks if ( this.credits == 'Senior' )
which will never be true because 'Senior' nor 'Junior' or any class is actually being saved to any variable in the current code- rather these class levels are just being returned to the console. Personally I think it's easiest to replace this if statement with one that uses the actual number of credits rather than class level. For example: if (this.credits > 60)
.
Additionally, to satisfy the challenge the else statement when if (this.credits > 60)
is FALSE needs to actually save 'none' as the value for this._major
rather than just return 'None' as a string.
For example, this code satisfies the challenge requirements for the set major() function:
set major(major) {
this._major = major;
if (this.credits > 60) {
return this._major;
} else {
this._major = 'none';
return 'this._major';
}
However, if you wanted to use if ( this.credits == 'Senior' )
(or Junior, etc) route. like you currently have, you probably could make that work as well, but earlier in the code the get level()
function only returned 'Senior', 'Junior', etc, rather than actually saved those values to a variable to be later used in the if statement in the set major() function. Like so:
get level() {
if (this.credits > 90 ) {
return 'Senior'; <!-- here -->
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
I'm guessing you'd have to create a class level variable, have the get level() function save 'Senior', 'Junior', etc to that variable, which could then be compared in the if statement you already have in your code.
Reminder though - if you want to go this route then remember that the OR operator needs to be complete on both sides! Like:
if (this.credits == 'Senior' || this.credits == 'Junior')
as opposed to;
if (this.credits == 'Senior' || 'Junior')
Hope this helps!
Marcus Alleyne
11,469 PointsMarcus Alleyne
11,469 PointsOoooh I can't believe I missed that! That makes a lot of sense Dylan Irvin! I totally forgot those class names were not saved to any variable! I was breaking my brain wondering why my if statement wasn't working. Thanks a lot for the help!