Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial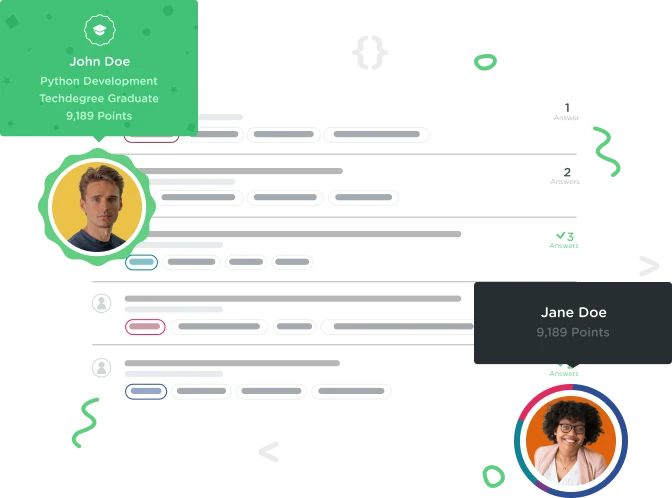

shane reichefeld
Courses Plus Student 2,232 Pointscreating a subclass
Any clarification to how its supposed to be?
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car {
override init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
var numberOfSeats: Int = 4
}
}
let someCar = Car(0,0)
1 Answer
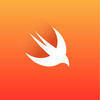
Steven Deutsch
21,046 PointsHey Shane Reichefeld,
Good work so far. Let's see if I can clear a few things up for you. First, to create a subclass of vehicle, you need to place a semicolon after the name of the new class declaration, followed by the class it is inheriting from.
class NewClass: ParentClass {
// class body here
}
Next, we just have to add a new property to this Car class, named numberOfSeats, with a default Int value of 4. Since the new properties you're adding all have default values, you don't need to implement another initializer, and you can inherit the initializer of the parent class. Therefore, our setup of this new subclass is relatively simple and complete.
We now need to create an instance of this class, and assign it to the constant someCar. We can do this by calling the initializer of the parent class. Since that initializer was declared using external argument names, withDoors and andWheels, we need to use them when calling the initializer.
class Vehicle {
var numberOfDoors: Int
var numberOfWheels: Int
init(withDoors doors: Int, andWheels wheels: Int) {
self.numberOfDoors = doors
self.numberOfWheels = wheels
}
}
// Enter your code below
class Car: Vehicle {
let numberOfSeats: Int = 4
}
let someCar = Car(withDoors: 4, andWheels: 4)
Good Luck