Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial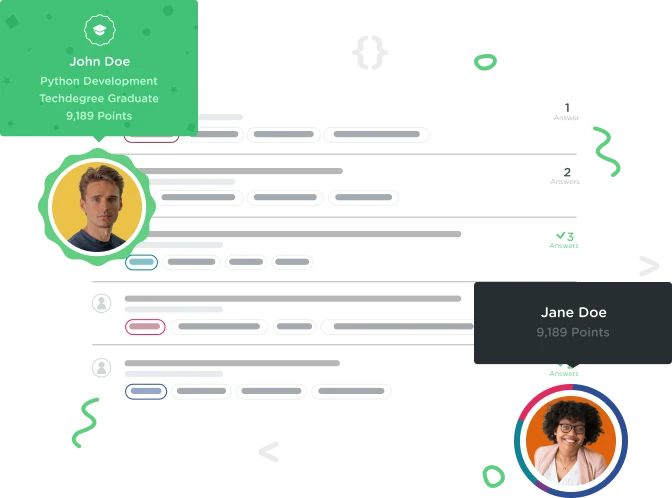
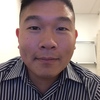
Yi Ren
4,990 PointsCreating an Array
What's the difference between:
array = Array.new => []
and
array = [] => []
It seems to me they are both doing the something, creating an new array. is there any reason that we should use the first one over the second one?
1 Answer
Shani Rivers
14,920 PointsThey are very similar, in that they create an array. Array = [] is a literal or just shorthand for Array = array.new, which is an array constructor that behind the scenes calls for the literal. The major advantage between the two is that using array.new will allow it to take arguments and a block.
Array.new # => [] or an empty array
Array.new(3) # => [nil, nil, nil], an array of size 3 with no values
Array.new(5, "yay") # => ["yay", "yay", "yay", "yay", "yay"]
# The folowing code creates a copy of an array passed in as a
# parameter with only one instance of the default object is
# created, this is where you can pass in a hash into an array, which
# is something you can not do with a literal
a = Array.new(2, Hash.new)
a[0]['dog'] = 'canine'
a # => [{"dog"=>"canine"}, {"dog"=>"canine"}]
a[1]['dog'] = 'Max'
a # => [{"dog"=>"Max"}, {"dog"=>"Max"}]
# Creating multiple instances of a[0]['dog'] = 'canine' by using the
# curly braces {}
a = Array.new(2) { Hash.new }
a # => [{"dog"=>"canine"}, {}
# The last thing you can do with an array constructor is give it a
# set size, in this case it's 5, and then calculate the element by
# passing the elementβs index to the given block and storing its return value.
squares = Array.new(5) {|i| i*i} squares # => [0, 1, 4, 9, 16]
copy = Array.new(squares) # initialized by copying squares[5] = 25
squares # => [0, 1, 4, 9, 16, 25]
copy # => [0, 1, 4, 9, 16]
Long story short, literals are prettier and will keep your code consistent, unless you are trying to do any of the above, then the constructor would be your best bet.
Hope that helps!
BTW: A lot of this info came from the book Programming Ruby and Stackoverflow... Google is your friend, my friend. :)
Yi Ren
4,990 PointsYi Ren
4,990 PointsThanks for the advice!
Shani Rivers
14,920 PointsShani Rivers
14,920 PointsNo problem! Thanks for asking, I learned something new too!