Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial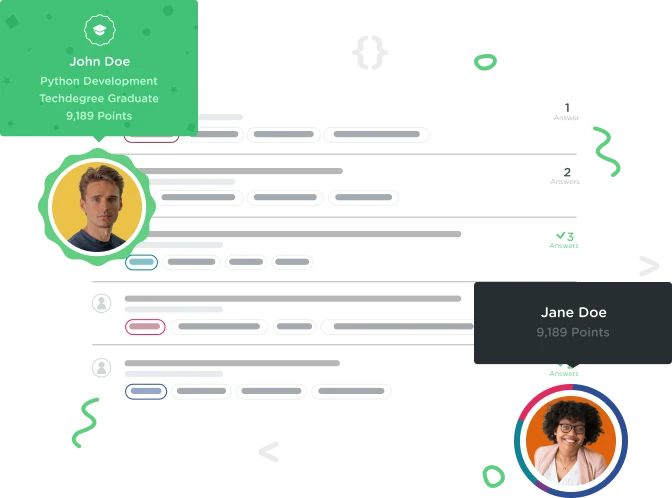
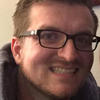
Michael Bates
13,344 PointsCreating and Appending Detached DOM Elements Challenge
I am having a difficult time solving this challenge, as I am having trouble understanding what needs to be done. What is the solution and reasoning behind it? Thanks in advance for any help!
//Create the Modal
var $modal = $("<div id='modal'></div>");
//Create a placeholder for text in the modal
var $placeHolder = $("<p id='placeHolder'></p>");
$modal.append($placeHolder);
//Create a button to dismiss modal and add it to modal
var $dismissButton = $("<button>Dismiss</button>");
//Hide modal when button is pressed
$dismissButton.click(function(){
$modal.hide(300);
});
$("body").append($modal);
//A function to show a modal
function displayModal(message) {
$placeHolder.text(message);
$modal.show();
}
//Show an example modal
displayModal("Hello World!");
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
<title>Modal Example</title>
</head>
<body>
<h1>Modal Example Page</h1>
<script src="//code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
3 Answers

Stuart Elmore
84 PointsHi Michael,
The reason you would want to create detached DOM elements is so that you can manipulate them with data before adding them to the page (this gives a better user experience by performing the manipulations 'off-screen' and not causing any disturbing flickering or shuddering).
It might also be because you want to load data from an external source before displaying it. For example; a Twitter-like feed could be loaded from an REST endpoint (or suchlike) and then you could construct the DOM markup in memory before appending it to the <body>
.
In the instance of the lightbox, you might want to do this in order to load a higher-resolution image off-screen and display it once you've loaded it completely, or maybe load from a Flickr feed asynchronously?
Let me know if you've got any further questions :)

Jacob Mishkin
23,118 PointsFirst, I would suggest that you post your attempt to solve the question, that way we can see what your thought process is for the logic. I will give you a hint though:
Check out the preview, the button is not in the div modal, maybe your should attach the button.
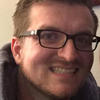
Michael Bates
13,344 PointsI am still having trouble with attempting a solution, which is why I haven't provided my own attempt at the answer. For some reason, I am totally lost at what to do.

Jacob Mishkin
23,118 PointsIts cool, look at the code, lets break it down:
questions to ask:
what is attached to the div modal? Answer: by looking at both the preview and code $placeholder is attached and showing.
why is that and not the button, what is different in the $placeholder code than $dismissButton? Answer: there is a jQuery method applied to $placeholder and not $dismissButton.
what is that jQuery method? Answer: its the append method. this method appends variables to other variables.
Do I want to append the $dismissButton to the div modal? Answer: yes.
I could just of given you the answer, its easy, the real thing you are learning here is 1. syntax, which can always be learned. 2. how to breakdown and solve a problem, this is the most important thing in these classes. The key is don't look at the big picture, breakdown the problem at its root then build up.
I hope this helps.
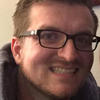
Michael Bates
13,344 PointsAhhhh.... That makes more sense now!! Thanks Jacob and Stuart! For some reason it just wasn't registering in my mind just shortly after watching the video. And when trying to do some of these code challenges, they can be hard to complete when done on an iPhone
Stuart Elmore
84 PointsStuart Elmore
84 PointsThe
$dismissButton
item is created separately to the rest of the modal so that it is easy to attach an event handler to it (see below)However, like Jacob said; it's not actually attached to anything before the modal window is attached to the DOM. Without wanting to answer it for you, you might want to explore how you can attach the
$dismissButton
. Bear in mind that detached DOM elements work (nearly) identically to attached DOM elements.