Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial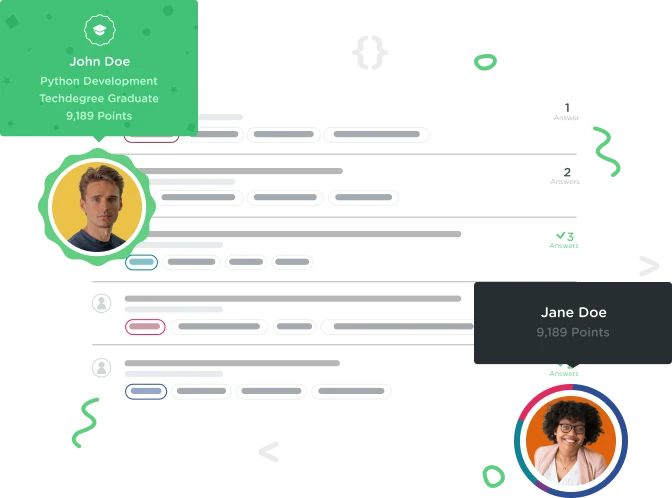

Robert Walker
17,146 PointsCreating dynamic pages for a set of PHP results.
making dynamic pages from results
The options open to me and how I would go about these different options.
I have a compare page you input a number of things and it then sends that information through POST,
compare.php > sends to results.php
From here PHP does all the magic stuff of comparing the inputs and the array / arrays output the compared results but once you close this page all the results are deleted and if you ever wanted to check the same results again you would need to go back to compare.php enter all the same details which would then send over POST var to the results.php again.
What I want to do is make unique pages every time you compare so instead of http://www.examplesite.com/results you would get something like:
http://www.examplesite.com/results?=1 or (Which I would pref if possible) http://www.examplesite.com/results/1.
So basically making it so every result set is stored so that people can have links to those specific results, I am not using a database and thought maybe I could store the result array / arrays in a text file and then when people typed in
http://www.examplesite.com/results/245
The normal results.php would be called but because the URL has/245 at the end it would then open that file and load output the saved results.
4 Answers
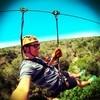
thomascawthorn
22,986 PointsThis is certainly possible, but not the most semantic way of creating output - remember DRY (Don't Repeat Yourself!). Although your html is only 86 lines, repeating all of your code in a new file for every single item would create a vast amount of html and php in time.
Any updates or changes you plan to make for this view would have to be changed in every single file (but this might be okay if you're only anticipating one or two files at any one time). It would be far more future-proof to create a template and pull in the specific data via an array/database.
You may find more success with your plan using these functions:
http://php.net/manual/en/function.file-exists.php
and
http://www.w3schools.com/php/php_file_create.asp
and
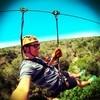
thomascawthorn
22,986 PointsHey man,
A really simple solution would be to use a database.. If you have any leeway on this, definitely use a database!
I'm not sure if you've seen the Shirts4Mike video series, but Randy first serves product information to the product page via an array in a single text file. There's probably not going to be too much in it either way but my guess is that an array would be faster to index than looking up a file, especially if this list is going to grow to quite an extensive length!
Are you adding in an expiry system? Otherwise that list could get super long :p
I can't tell if you're asking the question about the URL $_GET variables, but URL rewriting via htaccess is covered in the php application videos - Try the badge "Cleaning URLs with Simple Rewrite Rules" http://teamtreehouse.com/library/enhancing-a-simple-php-application-2

Robert Walker
17,146 PointsThe rewrite was what I needed for the URL but my biggest problem is the whole saving the page of results, I have lots of different arrays that contain parts of the information needed to output a full page of results.
I am struggling to see how to save all these outputs into a single file, is there a way to just save the entire outputted php and html into a single file?
Sort of:
if(isset($_GET['id'])){
//load results and output page from that file.
echo $savedfile;
die();
}else{
// continue with page normally / do all the processing from the POST
}
The main issues im having are:
How do I save these results uniquely, if someone hits "compare" how do I assign it a unique ID to that compare, to avoid two people hitting compare at the same time and possibly getting the same ID.
How do I save the outputted html and php into a single file. I have seen something about ob_start(); but not too sure how this works or if it would do what I need.
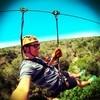
thomascawthorn
22,986 PointsAh, okay.
Here's the process I would take.. You will have to write the php part :p
Reading
In an include file, I would create the "Super Array". This would be a multidimensional array and hold each individual instances in child arrays. It would look something like:
$super_array[101] = array (
'first_name' => 'Tom',
'last_name' => 'Cawthorn'
)
$super_array[102] = array (
'first_name' => 'Robert',
'last_name' => 'Walker'
)
When you come to returning the information for each individual instance, you can call $super_array[$_GET], which will return a 2 dimensional array of the information you need to populate your page. I would turn this into a function because I absolutely love compartmentalising my code.
if (isset($_GET['id'])) {
$id = intval($_GET['id']);
$information = get_information($id);
//returns a child array of "Super Array". You can add this info to your page where required with $information[key];
} else {
//show prepared information / redirect
}
function get_information($id) {
//Maybe do some validation/combining of data
return $super_array[$id];
}
Writing You mentioned that incoming data is from a variety of arrays. No worries! When you create your new addition for $super_array, you can assign this info to the correct keys like this:
Create "Array" as multidimensional array - this will be the array to be 'saved' e.g.
$new_array['first_name'] = variable from array 1
$new_array['last_name'] = variable from array 2
Now you have all of your information in a single array, it is ready to be appended to $super_array. I would create another function called add_information($new_array).
There's one extra thing you'll need - the unique ID. The unique ID of $new_array will be +1 of the most recently appended array. Again, look to the documentation - but you might be able to do something with array_values() and count() etc to find the last $super_array value.
http://uk1.php.net/manual/en/function.array-values.php
http://uk1.php.net/manual/en/function.count.php
But essentially, you want to find the value for the final $super_array addition and add 1. You can double check it's unique by maybe validating with in_array($unique_id). If in_array returns true, the app will know the id is not unique and you can write some plan B code to sort it out.
http://uk1.php.net/manual/en/function.in-array.php
Now you have: 1) Array of new info 2) unique id
$super_array[$unique_id] = $new_arra
and now the info has been saved into your $super_array include file!
You should maybe think about adding a default timestamp to each item in $super_array and creating a function "remove_expired_items()" every time the array is used. You can call this function from any point in the process, but definitely something to consider!
This is just how I would do it.. I hope some of it helps
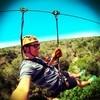
thomascawthorn
22,986 PointsI've just thought you will probably want to do all of this code and array finding before you show the results page. In which case, you should create an intermediate file to put this code in, and redirect with header("Location: results.php") to display the results to the user.

Robert Walker
17,146 PointsThanks Tom but this is a little too much work for the end result.
My html is only 86 lines and what I was hoping to do is take the html with the PHP generated HTML and storing them. then simply output again once the GET['id'] is given and valid.
Sort of like this:
//All my PHP code that processes the results and sorts everything.
Then my actual HTML
include header etc
outputted html from PHP
<div class="" style="margin-top: 50px">
<div class="large-6 small-6 columns" style="margin-top: 50px">
<div class="panel">
<a href="<?php echo $item['avatarfull']; ?>" alt="" title="" height="150" width="150"></img></a>
<a href="<?php echo $item['name']; ?></a>
</div>
<div class="" style="margin-top: 50px">
<div class="" style="margin-top: 50px">
<div class="panel">
<a href="<?php echo $item['avatarfull']; ?>" alt="" title="" height="150" width="150"></img></a>
<a href="<?php echo $item['name']; ?></a>
</div>
include footer etc
//========================================================================
What I am looking for is a way to just simple save the entire HTML after the php processing is done, that way I can save the entire html which of course would include the results the PHP processed and then when someone requested a saved file it would simple output all the HTML saved in that file.

Robert Walker
17,146 PointsThanks for all the help Tom.
Robert Walker
17,146 PointsRobert Walker
17,146 PointsI might not of been very clear there.
Lets say my $_GET['id'] is actually valid and has been requested by a user, IE: user types in a result set htttp://www.examplesite.com/result/1234
My PHP code would look like this:
Now what I want to do is this:
If its a first time compare I want the results page to run its course do all the magic and output the 86 lines of html with all the php results echoed in that.
Then I want to save just the HTML into a file, that way when someone requests this page again by doing http://www.examplesite.com/results/12345 it open the file and output the stored HTML in that file. The only PHP processing that would be required is:
Not sure if this makes sense hoping it does lol.
thomascawthorn
22,986 Pointsthomascawthorn
22,986 PointsIf your saved HTML is just page contents i.e. doesn't include a universal header / navigation etc, then this might work for you, and the functions I sent in my previous response should allow you to create, name and save a file with whatever content you pass into the argument.
You can just include the file you want by include("/myfiles/file" . $_GET );
I must stress that if you follow down this road and later want to make changes to the surrounding html or php, you will have to go through every file to do so! It is much better practice to save only the data and load the data into a template/view.
Don't make it too hard on yourself!