Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial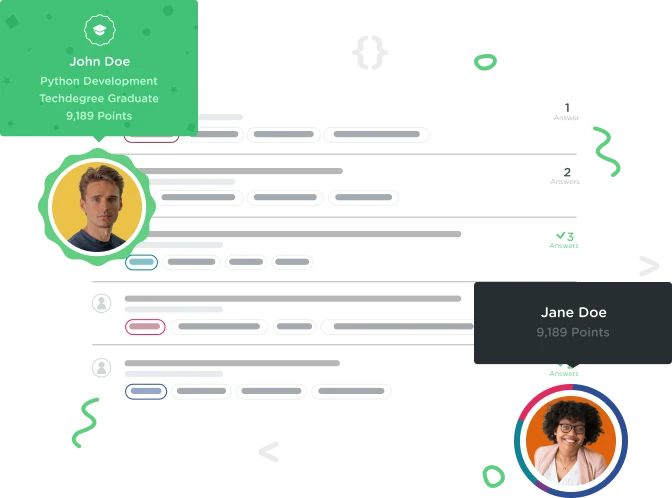
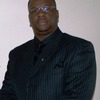
Roderick Stotts
7,884 PointsCreating Loops challenge 1 of 2
/Make a @for loop to iterate through the numbers 1 through 3. The for loop should create classes named in the format "item_#{$i}" and set the width to be $i * 100px./
.item {
width: 100%;
}
@for $i from 1 through 3 { .item:nth-child(#{$i}){ width: (100px * $i); } }
I am really confused what have I done wrong?
8 Answers
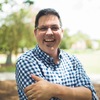
Dave McFarland
Treehouse TeacherYou're almost there Ben! When you're using a SASS variable you only need to surround the variable name with #{ }
when you're using that variable in a selector or property name. This is called interpolation. For example, in your code above, you must use the interpolation syntax to use the variable in a selector name:
.item_#{$i}
Say the variable $i is equal to 1, then SASS would spit out
.item_1
You don't need to use interpolation if you're just using the variable by itself or in an equation, for example when calculating the width of an element:
width: $i * 100px;
In other words, the final answer looks like this:
@for $i from 1 through 3 {
.item_#{$i} {
width: $i * 100px;
}
}
Technically, your answer is correct, SASS would convert the interpolated variable correctly. However, that won't pass the code challenge and it's best practice to only use interpolation when needed (to create selector or property values).
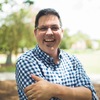
Dave McFarland
Treehouse TeacherYou're creating nth-child selectors (cool idea), but that's not what the code challenge is asking for. You want to end up with 3 separate classes like this
.item_#1 {
width: 100px;
}
.item_#2 {
width: 200px;
}
.item_#3 {
width: 300px;
}
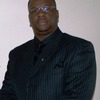
Roderick Stotts
7,884 PointsOk let me try some stuff.
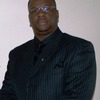
Roderick Stotts
7,884 PointsI don't know why I absolutely made it harder than it was, but I got it. Thank you Dave!
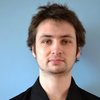
Ben Scholz
4,537 PointsI have written this code:
/* Write your SCSS code below. */
@for $i from 1 through 3 {
.item_#{$i} {
width:#{$i} * 100px;
}
}
And it doesn't work. Not sure where I went wrong. :/
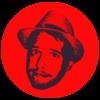
davpay
40,173 PointsYou've probably passed this by now but you have the variable wrapped in curly braces for the width, this passes:
/* Write your SCSS code below. */
@for $i from 1 through 3 {
.item_#{$i} {
width: $i * 100px;
}
}

Rolando Ponce de Le贸n
9,585 PointsGreat explanation Dave!
I was a bit confused at the beginning but your comment regarding interpolation helped me to see what i was doing wrong. I was enclosing the # sign inside parenthesis .item_(#{$i}) {
then I realized the was no need to use the parenthesis.
Thanks!
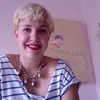
Elizabeth BABYCH
6,993 PointsI finally got this answer, but I don't understand why I had to specify the items and their widths, and then make a @for rule. Isn't this redundant?
.item_1 { width: 100px } .item_2 { width: 200px }
.item_3 { width: 300px; }
@for $i from 1 through 3 { item_#{$i} { width: $i * 100px; } }
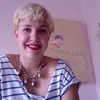
Elizabeth BABYCH
6,993 PointsA-ha, yes, yes it was redundant. I re-checked with your answer, tomp and it worked way better than mine. Defintely DRY-er.

inakicalvo
23,166 PointsThis worked for me:
@for $i from 1 through 3 {
.item_#{$i} {
width: $i * 100px;
}
}