Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial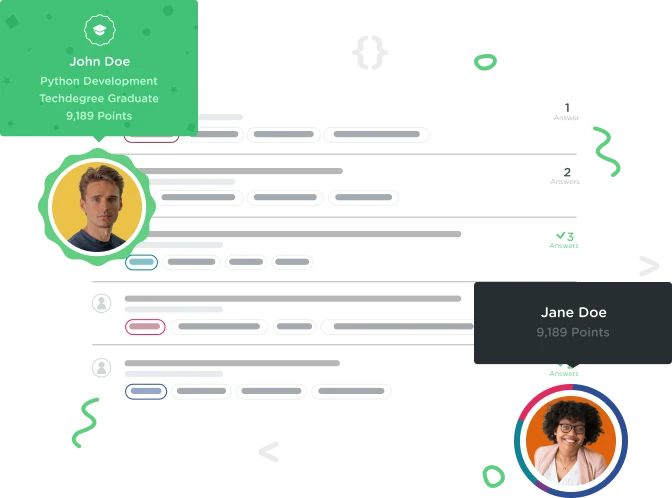
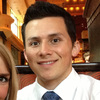
Jon Niu
2,843 PointsCreating methods
I feel a little sheepish, It's been like 10 minutes since my last question, but... Here's the request: Create a method on Fish named getInfo that takes no parameters and returns a string that includes the common_name, flavor, and record_weight for the fish. When called on $bass, getInfo might return "A Largemouth Bass is an Excellent flavored fish. The world record weight is 22 pounds 5 ounces."
And here's my code inside of the class: ''' function getInfo() { echo "A $name is an $flavor flavored fish. The world record weight is $record ."; } '''
Any wisdom to impart?
6 Answers
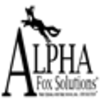
Shawn Gregory
Courses Plus Student 40,672 PointsHello,
What you have is correct (to a point). When you surround a phrase in double-quotes ("), you can inject any variable and it echos out the value inside of the variable. However, since this is a class method, you need to use the 'this->' in front of all of the properties in order to gain access to the classes properties. Just add the $this-> in front of your variables inside your return phrase and you'll be fine.
Cheers!
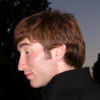
Eric Smith
3,537 PointsIn your getInfo method, similar to the example in the video, rather than echo, use return; you'll use echo below in its own statement to process the method with the properties from $bass.
Also, your concatenation is a bit off; make sure you know where to place the spaces and periods. Your line should be reading similar to the following:
public function getInfo(){
return "A " . $this->common_name . " is an " . $this->flavor . " flavored fish. The world record weight is " . $this->record_weight . ".";
}
Your echo statement then comes outside the class, just below your definition of $bass:
echo $bass->getInfo();

Juan P. Prado
69,294 PointsThanks Eric, also you can put all inside double quotes for readability
function getInfo(){
return "A $this->common_name is an $this->flavor flavored fish. The world record weight is $this->record_weight.";
}
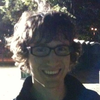
Ben Rubin
Courses Plus Student 14,658 PointsUse the . operator to concatinate strings with variables. Something like
echo 'A ' . $name . ' is an ' . $flavor // and so on
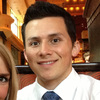
Jon Niu
2,843 PointsGot it. Thanks for the help.
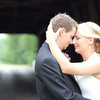
Jodie Whipple
11,321 PointsI am having a similar problem. I tried to follow the suggestions here but I'm still stuck. Here is what I have
<?php
class Fish { public $common_name; public $flavor; public $record_weight;
function __construct($name, $flavor, $record){ $this->common_name = $name; $this->flavor = $flavor; $this->record_weight = $record; }
public function getInfo(){ echo "A " . $this->common_name . "is an " $this->flavor . "flavored fish. The world record weight is " . $this->record_weight . "."; } }
$bass = new Fish("Largemouth Bass", "Excellent", "22 pounds 5 ounces");
?>
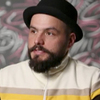
sergio verdeza
Courses Plus Student 10,765 PointsI don't seem to get it either :(
function getInfo() { return ("A " . $this->common_name . " is an " . $this->flavor . " flavored fish. The world record weight is " . $this->record_weight);

eamonamon
Courses Plus Student 2,863 Pointspublic function getInfo(){ return "A " . $this->common_name . " is an " . $this->flavor . " flavored fish. The world record weight is " . $this->record_weight . "."; }