Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial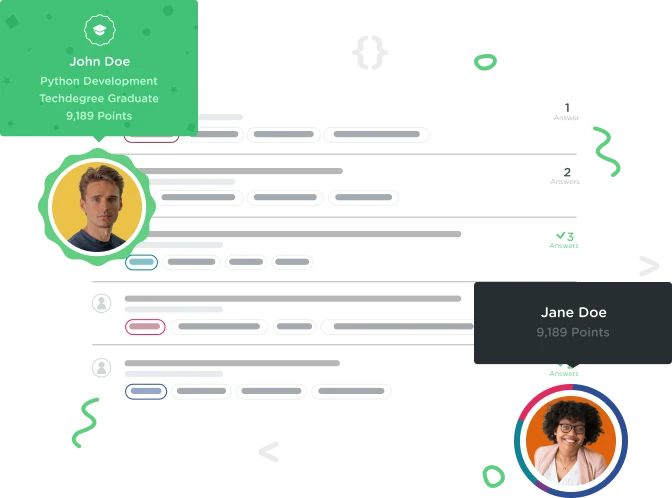
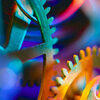
ja5on
10,338 PointsCreating New DOM Elements.
Does anyone feel this video is an uphill struggle... been here few weeks ago so I went away to learn more about CSS selectors, but still I'm stuck... just find hard to keep track of what Guil is doing, would be nice for some bite size material before this video...
3 Answers
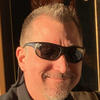
Peter Vann
36,425 PointsHey Jason!
I came up with this to help make callback functions a little easier to grasp:
const square = function(num) {
return num * num;
}
const quad = function(func, num) { // func is the callback function as a parameter (essentially a function variable)
return func(num) * func(num);
}
alert( quad(square, 3) ); // square is the callback function as an argument (an actual defined function)
And if you can't visualize it, in this case, the alert will display 81.
(3 * 3) * (3 * 3) === 9 * 9 === 81
And the thing that really makes it a callback function situation, is that, in this case, square() won't execute until quad() is called.
Does that make sense?
I hope that helps.
Stay safe and happy coding!
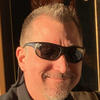
Peter Vann
36,425 PointsHi Jason!
I'll see if I can break this down into the "bite sized" material you would like.
First, are familiar with callback functions?
A callback function is basically a function passed into another function as an argument so that the function will execute at some future point (instead of immediately).
I recommend taking this course if you have not:
https://teamtreehouse.com/library/callback-functions-in-javascript
So consider this:
addItemButton.addEventListener('click', () => {
// code to be executed only when the button is clicked
});
() => {} is ES6 shorthand code for an anonymous (unamed) callback funtion.
So in the video, when the button is clicked a generic list-item element is created and stored in the li variable.
In other words, li = "<li></li>"; (effectively, just a string)
li.textContent = addItemInput.value puts whatever text that's typed in the input, so if "Jason" was typed in li would equal "<li>Jason</li>" (still effectively, just a string)
And at that point, as Guil states, li is still just existing in memory only - to see it on the page you will have to explicitly append it to the DOM for it to actually be part of the page HTML. (Content of the next video, I assume).
If you can't understand the decriptionP code from this explanation, lemmeeno, and I'll explain that, too!?!
More info:
https://www.w3schools.com/js/js_htmldom.asp
I hope that helps.
Stay safe and happy coding!
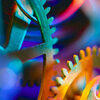
ja5on
10,338 PointsHey thanks for the reply, i used this in my code:-
button.addEventListener("click", function(){
p.innerHTML = input.value + ":";
});
Btw I didn't think callbacks were being used I thought the above I wrote or what you asked to consider were functions? I might be wrong... I think what i'm struggling is working out where everything is being used from like for example ID toggleList is changed to a string "toggleList" and thats put inside a variable then seems its used to be a name of a function then its used to get content from etc and when this happens several times to different items, I need 2 hours to study one 20 line document.
I tried callback function course I only managed alittle bit of it - very hard
Its keeping track of the variables and what's in them and what they being used for, plus guil goes quite fast. If I created and spent time on a project I would know what's happening all the while but with treehouse programs joining halfway through I'm not familiar with the plan ahead. - thats my struggle esp as coding is harder further in - still I treehouse is good at giving us the content so probably trying to recreate my own code along similar lines is best.
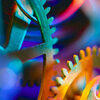
ja5on
10,338 Pointsconst square = function(num) {
return num * num;
}
So if I type square (5); the return is 25
const quad = function(func, num) {
return func(num) * func(num);
}
and here I type ? I'm guessing:- quad(square, 2); returns 32?
No I dont understand.
quad(quad, (2); returns error
two things go into parameters but one is func so I put function name then number but got error