Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial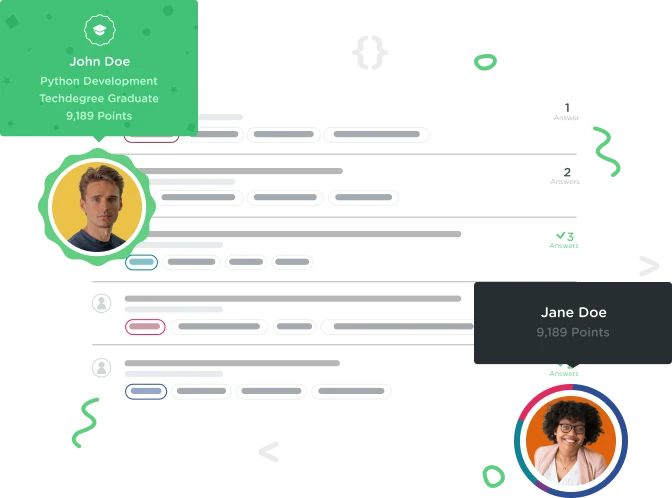
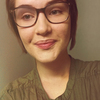
Pauliina K
1,973 PointsCreating new objects
I don't understand what I'm supposed to do in this exercise?
It says:
"Now that you've created the GoKart class. Please create a new GoKart object using the GoKart class, or blueprint. As you know it takes a single parameter, color when being built."
I don't understand anything..
Thanks in advance!
5 Answers
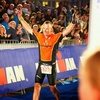
Steve Hunter
57,712 PointsTwo questions; I'll deal with them separately.
Yes, we write GoKart newKart because we are creating an instance of the GoKart class. The first part of the line, before the equals sign, allocates enough space in memory to hold a GoKart; it makes a GoKart-sized hole ready to be filled with an actual GoKart. To the right of the equals signed actually creates the GoKart itself. The equals sign puts that GoKart in the GoKart-sized hole. This is the two-step process called declaration and initialization. You declare a type of object then initialize it with an actual object. There are other names for this process that you'll come across. The second GoKart word is called a constructor. A constructor does what its name suggests! A constructor is always named the same as the class so, whilst we type the word
GoKart
twice, it is for very different reasons. One creates the room to put the object, the other makes the object.Using a method. Each class definition can contain various methods/functions that can be used on each instance of the class. The GoKart class has one called
getColor()
. This returns the color of the GoKart instance. In my example, it would return the string, "RED". So, calling the method on the instance (again, using my example) looks like:
newKart.getColor();
That returns a string which we want to print to the screen using the console malarkey, System.out.printf
. That printf
is a method which takes (among other things) a string as a parameter. It prints that string to the screen. Our newKart.getColor()
code returns a string. So, putting that all together you get:
System.out.printf(newKart.getColor());
That should complete the challenge.
Steve.
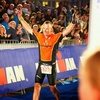
Steve Hunter
57,712 PointsHi Pauliina,
Rather than trying to explain this, I'll just show you. This is how you make a new object of the GoKart
class:
GoKart newKart = new GoKart("RED");
So, we first say we're making a GoKart and give it a name (any name - I chose newKart
). We then set that equal to a new GoKart
which is nice & easy! To create a new GoKart, the "constructor" wants to know what color it is, so we pass that in within the brackets.
Let me know if that doesn't make sense!
Steve.
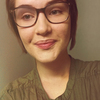
Pauliina K
1,973 PointsI think I understand! But why do we write GoKart newKart, and not String newKart or something similar? Is it because it's a new of -that- that we are making? But then again, we say new GoKart, so why do we need to write GoKart before too? Isn't that repeating? Wouldn't newKart = new GoKart ("blue"); work too?
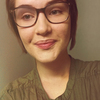
Pauliina K
1,973 PointsNow that I got to the next challenge, I don't get that either.. It says "Challenge Task 2 of 2 Print out using System.out.printf the color of the new object, using the getColor method."
I feel like I didn't quite understand this whole thing, and it's not that much talked about in the video?
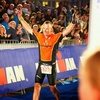
Steve Hunter
57,712 PointsHi Pauliina,
Let's start afresh on this challenge. Press F5 so you're right at the beginning - there are two steps.
The first part gives you a code block and asks you to Now that you've created the GoKart class. Please create a new GoKart object using the GoKart class, or blueprint. As you know it takes a single parameter, color when being built..
So you need to create a new GoKart
. That's like this:
GoKart newKart = new GoKart("RED");
So, we first say we're making a GoKart and give it a name (any name - I chose newKart
). We then set that equal to a new GoKart
which is nice & easy! To create a new GoKart
, the "constructor" wants to know what color it is, so we pass that in within the brackets.
OK so far?
The next task says: Print out using System.out.printf
the color of the new object, using the getColor
method.
For this, start on a new line, underneath your new GoKart
instance. As discussed this morning (or whatever time it is there, 09:28 here!), every instance of a GoKart
comes with features - getColor()
is one of them. Back in the code, update it to look like:
GoKart newKart = new GoKart("RED");
System.out.printf(newKart.getColor());
That adds the line needed to print out the color of newKart
.
Make sense? Let me know if not.
Steve.
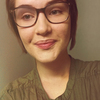
Pauliina K
1,973 PointsThank you so much! It worked now! And I sorta get it. Just a couple of things though:
- When do you use println and printf? What's the difference?
- How do you know when to put in parameters in a method? Like the getColor(), even though the parameters are empty. Do you always put () in methods?
- So, have I gotten this right? Getters, like getColor, and helpers, like isEmpty, are "built in", so they always work on classes? You don't have to define them or similar? Or..?
Also, I feel like I'm not understanding as much as I should. I'm doing this course, but that's it for now. I want to get in to a programming program at uni next autumn, but in this pace I'll never be good enough. How can I get better and understand more? I feel like everything I read just go in and out immediately. Nothing gets stuck unless I use it in an actual code, but I'm not good enough to code anything myself. My brain is kinda off lately due to stress, so that's why nothing makes sense anymore.. Very irritating.
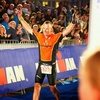
Steve Hunter
57,712 PointsOK - you're making progress - that's cool.
-
printf
andprintln
do similar things - I think one is called on the console object and the other isn't. I really wouldn't worry about the differences - they're academic and probably easily discovered with a quick Google. - All methods have brackets, yes. If the method taes no parameters, the brackets are empty, if the method does take a parameter, it'll throw an exception when you leave it out. ;-) Nah, seriously, the method signature will show you that it takes a parameter - we can use the getter & setter question, next, as an example.
- Getters and setters aren't built-in, no. You write them. The IDE (like Android Studio) will help you create them, but you do need to define them, unfortunately. Getters are pretty easy - they just return the private member variable, like
getColor()
returnedmColor
. Setters can be a little more complicated but the principle is identical - you pass a value in an it sets the appropriate value in the member variable. Let's usesetColor()
as an example - this shows you what a parameter looks like too:
public void setColor(String color){
mColor = color;
}
The member variable mColor
has private
access so only things inside the class can amend it. That's why we use getters and setters - it protects the member variable from changes as changes can only be made by the G & S. This method is a setter. It has public access, returns nothing (void
). It's called setColor
by convention. It takes one parameter which is a String
and inside the method, that string can be used with the variable named color
. That variable can be named anything and it doesn't matter what it is called when it is passed into the method - it just need to be of type String
.
So you could ave some code asking the user for their favourite colour so you can create a GoKart
for them.
//create a Kart for the user - unsure of colour
GoKart steveKart = new GoKart("DUNNO");
// ask for the colour
String favouriteColor = console.readLine("What is your favourite colour?: ");
// users answers "RED"
// call the setter
steveKart.setColor(favouriteColor);
That parameter, favouriteColor
goes into the setter method. Inside the setter method, the value contained in favouriteColor
is accessed by using the local name, color
and the privte member variable, mColor
, for the instance steveKart
is set to "RED".
public void setColor(String color){ // String color = favouriteColor
mColor = color; // sets mColor to color which is the same as favouriteColor
}
I'm good at making stuff over-complicated - I do hope that made some sense!!
Steve.
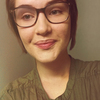
Pauliina K
1,973 PointsNo, but I think I get it. Thanks! Do you have any tips on how to get better at programming? Is it just practice, practice, practice? Should I repeat the courses I've already done in Java? Should I read books? Read code? Etc.
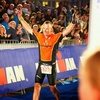
Steve Hunter
57,712 PointsI think that you're over estimating how much you should remember and under estimating how much you do remember. Learning a new language is really hard at first but it gets less challenging the more you do it. Everyone learns differently; some like to learn a load of languages in parallel as the common themes reinforce each of them - I get that. Others prefer to stick to one language - I get that too.
For me, it is all about repetition. Keep going over the concepts and trying to articulate them into English, rather than just code. I find that being in these forums really helps with that. Have a read at other people's posts in the same course you're in - see what responses say. Those responses will be articulating what I've written in this thread but in a different way. That way may suit you better - hearing the same message said in different contexts is a good way of getting it to sink in.
Keep going with the course on here. The Java stuff is quite heavy - Craig pushes you a lot in his courses which is great! Then try something on the Android course - that's all in Java too - that's a little less techy in approach but still a great set of courses taught by Ben. Just keep plugging away and asking in the COmmunity pages when you're having trouble. Also, look at other people's posts, like I suggested. You'll be answering posts in no time! But just being among the questions helps your brain iron out the bits you're not quite getting.
You'll make that course at Uni - just keep at it and try different elements of Java.
I hope that helps.
Steve.
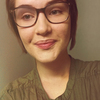
Pauliina K
1,973 PointsThank you again, Steve. I really appreciate the time you've taken to help me out. I feel like I'm finally back on track, and now things are going along much smoother! I will keep practicing and repeating things I don't fully grasp, I'll read a lot in the forum and I'm now taking notes on everything so that I can go back if I get lost and see how I described things. Things are making much more sense now. Thank you again! I think I needed a little kick in the butt.
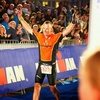
Steve Hunter
57,712 PointsCool - I'm glad you feel a little better about it. Keep up the good work!
And if you get stuck, you can always @ mention me in here and I'll come and see if I can help.
Steve.

Shezan Kazi
10,807 PointsHi Paulina,
Steve's answers are great and I'm glad we have mods like him. If you don't mind me asking, have you had programming experience prior to starting the java course? I actually did not and started JavaScript after Java. However, I feel like the fundamentals of programming are a lot easier to grasp when you start with JavaScript.
Even though JS and Java are not related, the principles applied in both languages are very similar and I find JavaScript easier to understand as it needn't be compiled, etc.
Cheers, Shezan
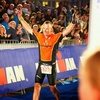
Steve Hunter
57,712 PointsI agree that the fundamentals of programming are common across pretty much all languages. Individuals may 'get along' better with some syntax over others, as you've found with JS. But coming across new concepts for the first time in whichever language can cause initial confusion often caused by a lack of confidence or familiarity.
Repetition remains a good way of embedding new skills; hoping to remember concepts after just one pass is a challenge. Revisiting the same, or similar, concept in a different context such as a different language, is a great way to solidify the foundation of that knowledge.
Steve.
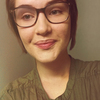
Pauliina K
1,973 PointsI have no prior programming experience. I've tried JavaScript, just for a couple of hours, before I started with Java. But, like Steve says, it just confused me. I mixed up all the different words etc. Plus, I wont need JavaScript for anything as I truly hate making webpages and web-based things. Java makes more sense to me. So, I'm just gonna keep practice Java for now!
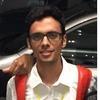
Christiaan Quyn
14,706 PointsExactly my thoughts coming into the challenge. It was a tough one and the tasks completely boggled my mind. I'm still having a tough time grasping the concept of Objects and Classes but hopefully with time and repetition I'm hoping I'll get to where I want to be :) Great Answers Steve !
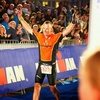
Steve Hunter
57,712 PointsHi Christiaan,
The concepts can seem challenging at first. But, as you say, if you work with them they will become familiar and you'll grow your understanding. These concepts are cross-language so they are transferable in other language than Java. The way the code is written may be slightly different but the concept of classes and instance/objects are the same.
Keep on working your way through it and ask questions in the Community pages if you get stuck.Trying to articulate a question forces you to think about what you know and where your knowledge gap is. That really helps solidify your thoughts.
Enjoy your courses. :-)
Steve.
Pauliina K
1,973 PointsPauliina K
1,973 PointsThank you for the thorough explanation! It helps a lot! One thing though, how can getColor work when I haven't defined Color anywhere in this code? (We did in the previous challenges, but it's not visible now, so maybe it's there, but not visible?) I understand that 'get' always works, but how can getColor work? I mean, how can the computer know what it's supposed to 'get'? If you understand what I mean?
Pauliina K
1,973 PointsPauliina K
1,973 PointsAlso, I haven't defined newKart or similar in the visible code, so I get this error:
./Example.java:6: error: cannot find symbol
symbol: variable newKart location: class Example 1 error
I don't understand where and how to define it. Do I just make a string, or do I have to make a public class with it? Or..?
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHi Pauliina,
The
getColor()
method will work because the compiler you are working with knows what theGoKart
class can do. So, once you've declared an instance of aGoKart
, all its methods are available to that instance. So, inside ofGoKart
there's a method called (and it could be called anything, but it is good practice to name the getters and setters in this way)getColor
that just returns the member variable called, probably, something likemColor
.Something like that. So your instance of
GoKart
comes loaded with this functionality.I'm not sure why you're getting the cannot find symbol error. The first part of this challenge was to define a new object of a
GoKart
. I called itnewKart
, did you call it something else?You initial line of code should have something like:
GoKart newKart = new GoKart("RED");
Do you have that in there?
Steve.
Pauliina K
1,973 PointsPauliina K
1,973 PointsNo, I wrote that code in the previous challenges and now this is all the code that's there:
public class Example {
The exercise says: "Now that you've created the GoKart class. Please create a new GoKart object using the GoKart class, or blueprint. As you know it takes a single parameter, color when being built."
So, do I need to re-write the old code? This is the code I have in the previous challenge:
public class GoKart { private String mColor = "red"; public String getColor() { return mColor; } }
I feel so lost. Everything was going great until this challenge came along, and now nothing makes sense anymore.