Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial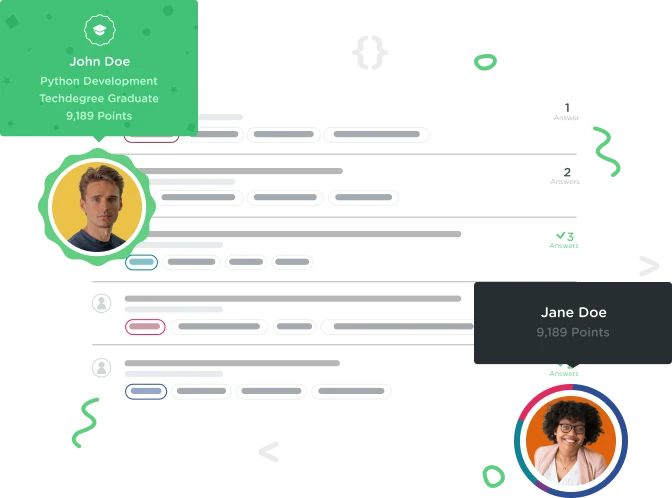
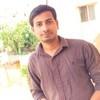
RUPESH BASUDE
2,932 Pointscreating Prototype
We're adding a new method to every instance when a Monster is created. Use a prototype instead for the takeDamage method. Can anyone tell me how to solve this?
function Monster(name) {
this.name = name;
this.health = 100;
Monster.Prototype.takeDamage = function (){
this.health--;
}
}
3 Answers
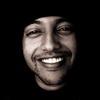
miikis
44,957 PointsHello Rupesh,
You've almost got it. It's a syntax error, mostly. Here's how it should look:
//This is the constructor for the Monster object
//Everything in here is dependent on every new instance of the Monster object
//For example: var cookieMonster = new Monster("Sid") would instantiate a new Monster object whose NAME property would have the value of "Sid" and whose HEALTH property would have the value of "100"
function Monster(name) {
this.name = name;
this.health = 100;
}
//This is a method for every Monster object ever to be instantiated
//Rather than include it in the constructor, we include it on the constructor's PROTOTYPE property
//This is so that you don't need to create a new version of this method for every Monster instantiation — instead every MONSTER instantiation is given access to the PROTOTYPE property
Monster.prototype.takeDamage = function() {
this.health--;
}
A while back, when I was first learning JavaScript, I asked a similar question here about prototypes. Dave McFarland gave a pretty apt answer. You should check it out.
William Li
Courses Plus Student 26,868 PointsHi there, couple issues here.
- the word Prototype is misspelled, it needs to be in all lower caps.
- the implementation of prototype should be moved outside of the Monster function
function Monster(name) {
this.name = name;
this.health = 100;
}
Monster.prototype.takeDamage = function (){
this.health--;
}
Hope it helps.
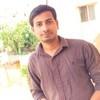
RUPESH BASUDE
2,932 PointsThank you very much. actually i did not understand the prototype concept clearly. in the previous video, why & how should we call one method function from another method
var dice=new dice(6);
var dice10=new dice(10);
Here we are creating the instance of "dice" function constructor.then why we calling with "dice10" method? its not there in the program and how it is possible?
William Li
Courses Plus Student 26,868 PointsHi, Rupesh, I'll try to answer your question the best I can.
In Andrew's original code during the lecture.
function Dice(sides) {
this.sides = sides;
this.roll = function () {
var randomNumber = Math.floor(Math.random() * this.sides) + 1;
return randomNumber;
}
}
This is the constructor function, you may think of it as blueprint for creating new object; in this particular constructor function, each object it creates has an attribute -- this.sides
and a method -- this.roll()
. Now, attribute and method are just some fancy terms programmer use in OOP, if they sound confusing, just think of attribute as variable attaches to an object, whereas method as function attaches to an object.
With a constructor function properly defined, we can create any number of new objects from it using the new statement.
var dice=new dice(6); // create a new instance of object named dice
var dice10=new dice(10); // create another instance of object named dice10
Now, to answer your question.
Here we are creating the instance of "dice" function constructor.then why we calling with "dice10" method? its not there in the program and how it is possible?
No, we're not calling "dice10" method. Both dice and dice10 are instances of objects created using the constructor function function Dice(sides) { ...}
. You can confirm this by outputting the sides attributes from them.
console.log(dice.sides); // => 6
console.log(dice10.sides); // => 10
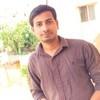
RUPESH BASUDE
2,932 PointsI have one more doubt.that is, This are the lines i have copied from the linked article of Dave sir by you
"By placing the roll() function on the prototype, you keep each instance free of duplicated code. roll() is on the prototype but not in each instance, so the function code is not duplicated each time a new Dice is created. Those individual instances simply look at the prototype and run the function on it. This saves memory."
Here, how can it keep free of duplicated code? and where & how the duplication comes into picture if we didn't mention "prototype"