Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial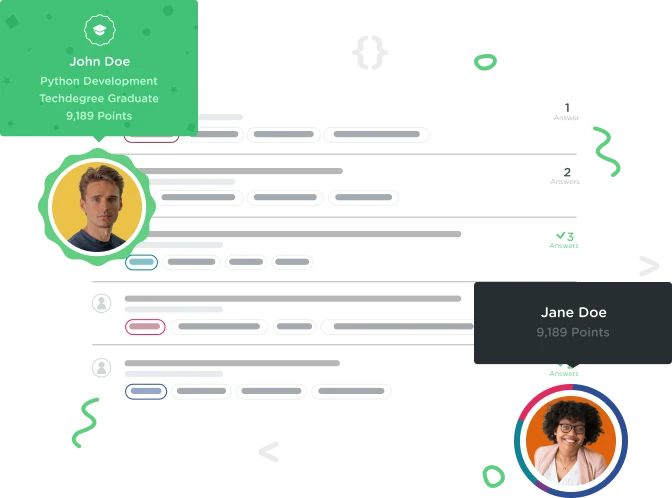
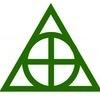
Daniel Bateman
13,268 PointsCreating Setters code challenge
Asked this in another question, but didn't get an answer that helped me to solve my problem.
The code challenge states: "Inside the major() setter method, set the student's major to a backing property "major". If the student's level is Junior or Senior, the value of the backing property should be equal to the parameter passed to the setter method. If the student is only a Freshman or Sophomore, set the "major" backing property equal to 'None'."
It keeps on returning "unexpected identifier". I have run the code with "this.major" and "this._major" and both return the same error.
Any thoughts?
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
set major(major) {
if (this.level === 'Senior' || this.level === 'Junior') {
this.major = major;
} else {
this.major = 'none';
}
}
}
}
}
var student = new Student(3.9, 60);
3 Answers

Nathan Angulo
4,565 Points''The name of a property can never be the same name as the name of a getter or setter method''
"We have to create a property name that's different from major to hold the value of major"
...which is why I used the backing property naming convention _major. I think the underscore identical name thing will make more sense the more we use it.
The get major() method that I used in my code wasn't necessary for this practice, but if we wanted to get the value of the backing property we could use it.
Hope this._helps!
class Student {
constructor(gpa, credits){
this.gpa = gpa;
this.credits = credits;
}
stringGPA() {
return this.gpa.toString();
}
get level() {
if (this.credits > 90 ) {
return 'Senior';
} else if (this.credits > 60) {
return 'Junior';
} else if (this.credits > 30) {
return 'Sophomore';
} else {
return 'Freshman';
}
}
get major() {
return this._major;
}
set major(major) {
this._major = major;
if(this.level === 'Senior' || this.level === 'Junior') {
this._major = major;
}
else {
this._major = 'None';
}
}
}
var student = new Student(3.9, 60);
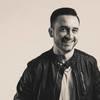
Chris Pulver
7,624 PointsUnexpected identifier typically means you are missing or have too many of something like curly braces, parentheses, etc. Otherwise, you are on the right track.

Caleb Campbell
5,747 Pointsok, so my answer looks almost identical to Nathan's but Treehouse responds with "Maximum call stack size exceeded" and it will not let me pass the challenge.
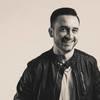
Chris Pulver
7,624 PointsCan you post your code so we can troubleshoot?
Caleb Campbell
5,747 PointsCaleb Campbell
5,747 Pointsso, out of curiosity, "this._major = major;" that sits just under the if statement, why is major not within quotes but the word none that follows it is? and why did the exercise accept it?
Chris Pulver
7,624 PointsChris Pulver
7,624 Points" 'None' " is a string value, while "major" will change depending on the value of the argument that would be supplied to the function.