Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial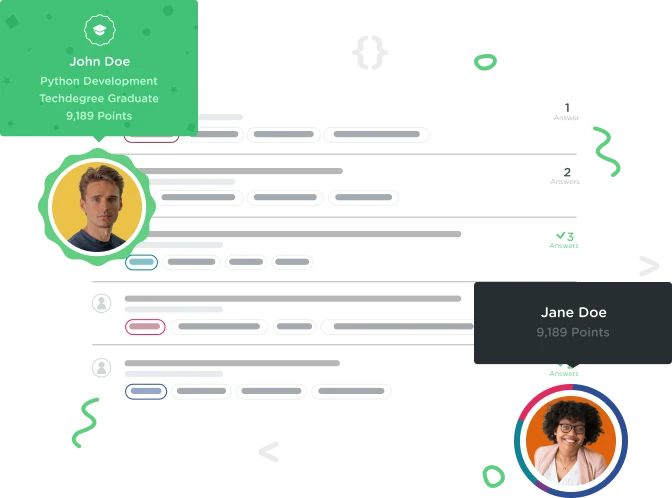
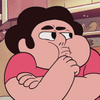
Ludwing Najera
4,596 PointsCreating time int value error
I was creating a time int value for my weather app when Xcode spat out an error saying "'Int' is not convertible to 'String'"
if you have no idea what i'm talking about, watch the video first.
here's my code:
import Foundation
struct Current {
var currentTime: String
var temperature: Int
var humidity: Double
var precipProbability: Double
var summary: String
var icon: String
init(weatherDictionary: NSDictionary){
//initialization
let currentWeather = weatherDictionary["currently"] as
NSDictionary
let currentTimeIntValue = currentTime = currentWeather["time"] as Int
currentTime = dateStringFromUnixTime(currentTimeIntValue)
temperature = currentWeather["temperature"] as Int
humidity = currentWeather["humidity"] as Double
precipProbability = currentWeather["precipProbability"] as Double
summary = currentWeather["summary"] as String
icon = currentWeather["icon"] as String
}
func dateStringFromUnixTime(unixTime: Int) -> String {
let timeInSeconds = NSTimeInterval(unixTime)
let weatherDate = NSDate(timeIntervalSince1970: timeInSeconds)
let dateFormatter = NSDateFormatter()
dateFormatter.timeStyle = .ShortStyle
return dateFormatter.stringFromDate(weatherDate)
}
}
3 Answers

Martin Wildfeuer
Courses Plus Student 11,071 PointsHey there,
One problem is that you are downcasting optionals without unwrapping them:
// weatherDictionary["currently"] returns an optional,
// as key and/or value might not exist
let currentWeather = weatherDictionary["currently"] as NSDictionary
The quick and dirty solution is to force unwrap the value with as!
let currentWeather = weatherDictionary["currently"] as! NSDictionary
...
The downside: if your dictionary is missing one of these values, it will crash.
Moreover, you cannot call a method of the struct you are initializing before all stored properties are set, Xcode will alert you about that. That is why I have extended NSDate
with your unixTime function:
struct Current {
var temperature: Int
var humidity: Double
var precipProbability: Double
var summary: String
var icon: String
var currentTime: String
init(weatherDictionary: NSDictionary){
let currentWeather = weatherDictionary["currently"] as! NSDictionary
temperature = currentWeather["temperature"] as! Int
humidity = currentWeather["humidity"] as! Double
precipProbability = currentWeather["precipProbability"] as! Double
summary = currentWeather["summary"] as! String
icon = currentWeather["icon"] as! String
currentTime = NSDate.dateStringFromUnixTime(currentWeather["time"] as! Int)
}
}
extension NSDate {
class func dateStringFromUnixTime(unixTime: Int) -> String {
let timeInSeconds = NSTimeInterval(unixTime)
let weatherDate = self(timeIntervalSince1970: timeInSeconds)
let dateFormatter = NSDateFormatter()
dateFormatter.timeStyle = .ShortStyle
return dateFormatter.stringFromDate(weatherDate)
}
}
There might or might not be better ways of implementing this, it is just a quick draft of how to make existing code work. Hope that helps :)
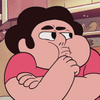
Ludwing Najera
4,596 Pointsplease don't say the problem is that i'm using the retired course because i would really not like to start my app all over again
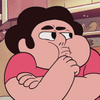
Ludwing Najera
4,596 Pointswhen i made the adjustments, Xcode spat out an error saying "Expected type after 'as'"
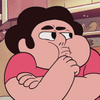
Ludwing Najera
4,596 PointsOh... Xcode added in optional '!' for some reason, but i removed them, And now it works fine! Thanks Martin!