Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial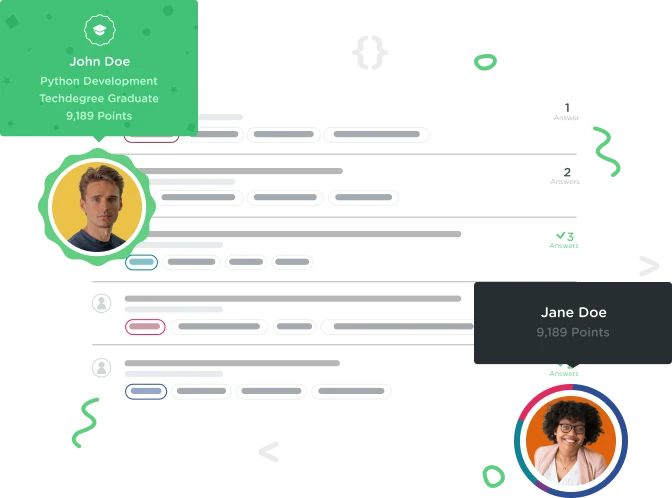
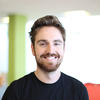
Kristian Woods
23,414 PointsCreating variables inside of loops and creating loops outside of loops
Looking to figure out if when you create a variable inside a loop, whether it resets the value of the variable after each iteration. This is opposed to creating a variable OUTSIDE of a loop, but including it within the loop.
Does the loop that is created OUTSIDE the loop retain the value from each iteration?
//var total = 0;
//
//
//
//for (var i = 1; i <= 5; i++) {
// var coffeeType = prompt("What would you like to drink?");
// var coffeePrice = parseInt(prompt("What is the price?"));
// total += coffeePrice;
// document.write("<p>your chosen drinks are " + coffeeType + " and the price is " + coffeePrice + "</p>");
//
//}
//
//document.write("<p>total price is " + "£" + total + "</p>");
By creating a new variable each time the loop runs, it resets the previous variable, so that each value isnt added to the next. THIS IS WHY ITS IMPORTANT TO USE THE "VAR" KEYWORD - SO THAT THE VAR RESETS EACH TIME THE LOOP RUNS.
example: by creating the variable "coffeeType" OUTSIDE the loop, then including that variable WITHOUT THE VAR KEYWORD INSIDE the loop, it then continues to add each new value. so by including the var keyword, the loop flushes out the previous value:
var total = 0;
for (var i = 1; i <= 5; i++) {
var coffeeType = prompt("What would you like to drink?"); // this variable WILL reset each time
var coffeePrice = parseInt(prompt("What is the price?")); // this variable WILL reset each time
total += coffeePrice; // this variable will continue to add each value each time the loop is run
document.write("<p>your chosen drinks are " + coffeeType + " and the price is " + coffeePrice + "</p>");
}
document.write("<p>total price is " + "£" + total + "</p>");
opposed to
//var total = 0;
//var coffeeType;
//
//for (var i = 1; i <= 5; i++) {
// coffeeType = prompt("What would you like to drink?"); // this variable WON'T reset each time
// coffeePrice = parseInt(prompt("What is the price?")); // this variable WON'T reset each time
// total += coffeePrice;
// document.write("<p>your chosen drinks are " + coffeeType + " and the price is " + coffeePrice + "</p>");
//
//}
//
//document.write("<p>total price is " + "£" + total + "</p>");
if this is the case, can someone explain why?
Thanks
1 Answer
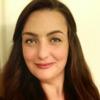
Jennifer Nordell
Treehouse TeacherWhat you're describing here is what we refer to as "scope". If you declare a variable inside a loop (or function), then that variable will only be accessible inside that loop/function. If you create it outside, then you can adjust the values inside the loop/function and the variable outside will retain that information (assuming you don't redeclare the variable somewhere else).
Here's a simple example using a function:
function squared(x) {
return x * x;
}
var y = squared(8);
console.log(y); //This will log 64 to the console
console.log(x); //This will generate an error because x does not yet exist in this "scope"
edited for additional note
And yes, every time you declare a variable you are resetting its value (assuming it is in the same scope). Here's some MDN documentation on variable scope. https://msdn.microsoft.com/library/bzt2dkta(v=vs.94).aspx
Hope this helps!