Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial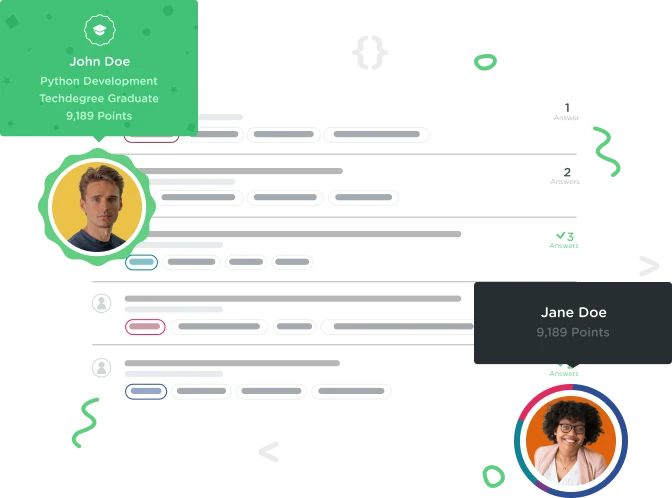

Patrick Iwanicki
2,178 PointsCredit Card Input field with Icon Selector
https://secure.karelia.com/buy_now/
Looking to replicate the functionality seen here where CC icon becomes focused with digit input
Any thoughts???
5 Answers

Joseph Cahill
Courses Plus Student 4,739 PointsTry this:
document.write('3400 0000 0000 009=' + GetCreditCardTypeByNumber('3400 0000 0000 009'));
document.write('6011 0000 0000 0004=' + GetCreditCardTypeByNumber('6011 0000 0000 0004'));
document.write('5500 0000 0000 0004=' + GetCreditCardTypeByNumber('5500 0000 0000 0004'));
document.write('4111 1111 1111 1111=' + GetCreditCardTypeByNumber('4111 1111 1111 1111'));
function GetCreditCardTypeByNumber(ccnumber) {
var cc = (ccnumber + '').replace(/\s/g, ''); //remove space
if ((/^(34|37)/).test(cc) && cc.length == 15) {
return 'AMEX'; //AMEX begins with 34 or 37, and length is 15.
} else if ((/^(51|52|53|54|55)/).test(cc) && cc.length == 16) {
return 'MasterCard'; //MasterCard beigins with 51-55, and length is 16.
} else if ((/^(4)/).test(cc) && (cc.length == 13 || cc.length == 16)) {
return 'Visa'; //VISA begins with 4, and length is 13 or 16.
} else if ((/^(300|301|302|303|304|305|36|38)/).test(cc) && cc.length == 14) {
return 'DinersClub'; //Diners Club begins with 300-305 or 36 or 38, and length is 14.
} else if ((/^(2014|2149)/).test(cc) && cc.length == 15) {
return 'enRoute'; //enRoute begins with 2014 or 2149, and length is 15.
} else if ((/^(6011)/).test(cc) && cc.length == 16) {
return 'Discover'; //Discover begins with 6011, and length is 16.
} else if ((/^(3)/).test(cc) && cc.length == 16) {
return 'JCB'; //JCB begins with 3, and length is 16.
} else if ((/^(2131|1800)/).test(cc) && cc.length == 15) {
return 'JCB'; //JCB begins with 2131 or 1800, and length is 15.
}
return '?'; //unknown type
}
function IsValidCC(str) { //A boolean version
if (GetCreditCardTypeByNumber(str) == '?') return false;
return true;
}
Source: http://javascript.gakaa.com/credit-card-type-by-number.aspx

James Barnett
39,199 PointsYou can do that with Javascript.
Rules for detecting credit cards are extermely simple:
- Visa: start with
4
- MasterCard: start with
51
-55
- American Express: start with
34
or37
Here's a great demo of detecting credit card types with Javascript
Here are test numbers for all major credit cards
This post on auto detecting credit card types with Javascript should get you started.
Just remember under no circumstance should you be storing credit card numbers, use a merchant gateway service like Stripe instead, they do all the hard work of for you.
Another option is Braintree which is used by Treehouse to process all of your credit card payments everymonth.

Patrick Iwanicki
2,178 PointsFound some good stuff here as well: http://webstandardssherpa.com/reviews/auto-detecting-credit-card-type/

James Barnett
39,199 Points@Patrick - Great minds think alike, that's the post I linked to.
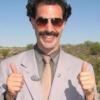
Keith Wolf
1,191 PointsPretty neat functionality on the site linked by the OP,,, start typing 34 or 37 (for amex) and it flips the CCD picture for amex. Very thoughtful.