Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial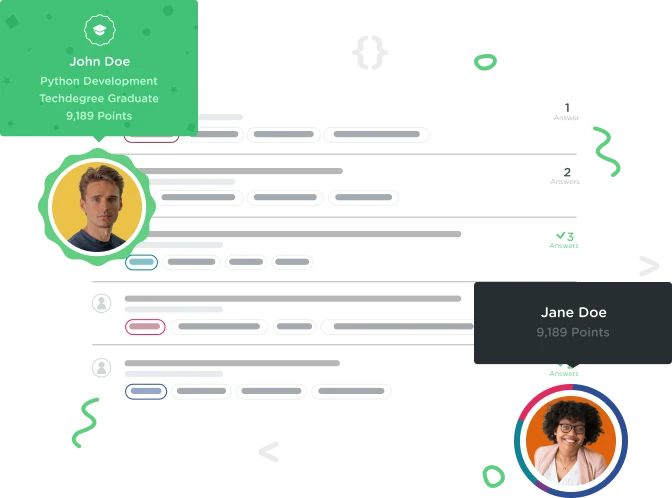

Robert Torres
2,736 PointsCredit Card sum challenge.
Contain all variables and code needed within a function. Have that function take one argument which will be an array of credit card number strings. Determine the sum of digits for each credit card number. Determine which credit card number has the last largest sum of digits. Use a return statement to return the required card number in itsβ original form.
I pretty much have this, just need to return the original form of the card.
var reducedCards = [];
console.log(reducedCards)
function printNumbers(arrayOfCards) {
arrayOfCards.forEach(function(card){
card = card.replace(/-/g, '').split('').reduce(function(prev, curr){
return Number(prev) + Number(curr);
});
reducedCards.push(card);
});
console.log(Math.max.apply(Math, reducedCards));
}
printNumbers(cards)
1 Answer

Thomas Nilsen
14,957 PointsFirst - a few notes.
This line:
card.replace(/-/g, '').split('')
is unnecessary. You can just split it directly, like this:
card.split('-')
Here is the code:
var cards = ['9999-9999','1212-1212','2323-2323','3434-3434'];
function getLargerstCreditCard(arrayOfCards) {
/*
1) This just essentially makes a new 2D array, with the original card-form and it's sum, like this:
[ [ '9999-9999', 19998 ],
[ '1212-1212', 2424 ],
[ '2323-2323', 4646 ],
[ '3434-3434', 6868 ] ]
2) The sort method just sorts it by the sum desc (largest sum first)
3) the [0][0] in the end is basically get the first credit-card number in the 2D array
*/
return arrayOfCards
// 1 - see comments above
.reduce((a,b) => {
a.push([b, b.split('-').map(Number).reduce((a,b) => a+b)]);
return a;
}, [])
//2
.sort((a,b) => b[1] - a[1])[0][0];
}
console.log(getLargerstCreditCard(cards))
Robert Torres
2,736 PointsRobert Torres
2,736 PointsOh yeah this is a great example! Much cleaner than the other two versions I was trying to work through. I pretty much know whats going on in this code. Thank you sir!