Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial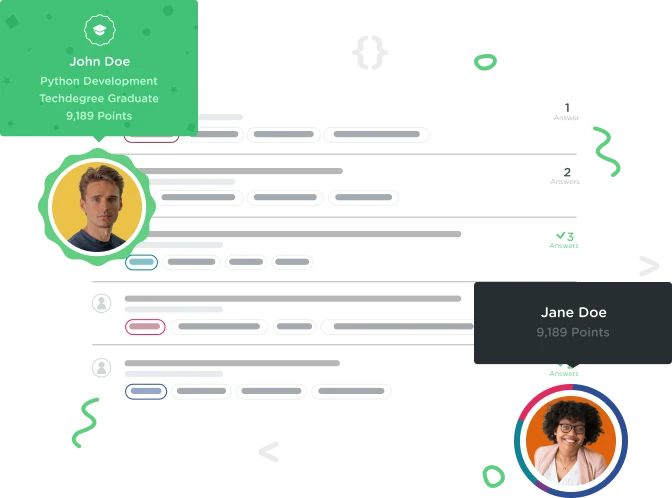

Sebastiaan Stoffels
3,075 PointsCritique my disemvowel
Hi all, This code worked, and i passed the test, but I think it could still be better as I didn't use .upper or .lower.
Keen for a mod, or someone more experienced to help me understand if my brain is working in the correct way to address this kind of problem:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
word_as_list = list(word)
for vowel in vowels:
for letters in word_as_list:
try:
word_as_list.remove(vowel)
except ValueError:
pass
return ''.join(word_as_list)
1 Answer
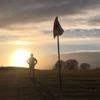
Stuart Wright
41,118 PointsYou can run into problems when you try to loop over a list and remove items from it as you go. Although it seems to have worked in this case, it's generally bad practice. It would be safer to create a new output variable, something like:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
word_as_list = []
for letter in word:
if letter not in vowels:
word_as_list.append(letter)
return ''.join(word_as_list)
I wouldn't worry too much about using .upper() or .lower() for this challenge as it doesn't actually save any work (it's just as quick to type out the full list of vowels), but here is an example of how you could have worked it in:
def disemvowel(word):
vowels = ["a", "e", "i", "o", "u"]
word_as_list = []
for letter in word:
if letter.lower() not in vowels:
word_as_list.append(letter)
return ''.join(word_as_list)