Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial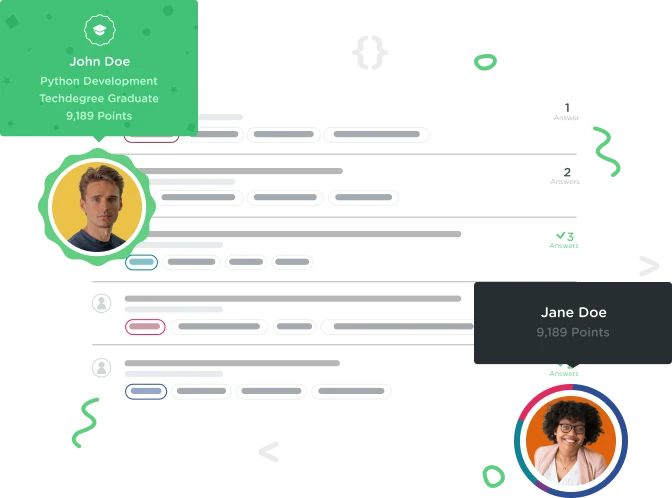
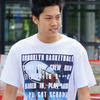
Dave Six
8,366 PointsCrossfade Images in List
I'm trying to fade in images on click on a text. My problem is that I don't know how to cycle through the images.
the HTML looks like this:
<div class="flexslider">
<ul class="slides">
<li><img src="img_1.jpg"></li>
<li><img src="img_2"></li>
<li><img src="img_3"></li>
</ul>
</div>
The script is almost working except that it's just fading once
jQuery(function(){
jQuery('.flexslider .slides li:gt(0)').hide();
setInterval(function(){
jQuery('.flexslider .slides li:first-child').fadeOut()
.next('li').fadeIn() },
3000);
});
I also tried changing the Selector on line 4 without the first-child but I did not work as expected since it's fading directly to the last image and just repeating fading in the last image.
I hope you guys can help me out a little.
Dave
2 Answers
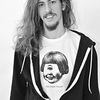
eck
43,038 PointsTry creating a class, maybe something like "active-slide", and placing it on the first slide you want to activate. You can then use this class to in your selector and when it is time to switch to a new slide you can remove the class and add it to the next element.
Here is an example (untested code):
jQuery(function(){
jQuery('.flexslider .slides li').hide();
jQuery('.flexslider .slides li.active-slide').show();
setInterval(function(){
jQuery('.flexslider .slides li.active-slide').fadeOut().removeClass("active-slide").next('li').addClass("active-slide").fadeIn() },
3000);
});
Hopefully it is relevant to your question and helpful :D
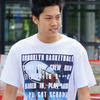
Dave Six
8,366 PointsHey Erik,
thanks a lot for your reply! The code is working just fine! Thanks again mate!
Dave
eck
43,038 Pointseck
43,038 PointsI gave it a test run, and the following js does loop properly.