Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial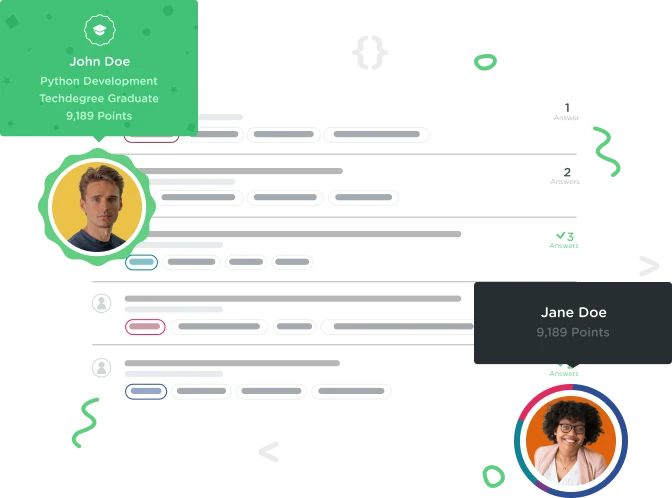

Robert Shaw
1,567 PointsCrystal Ball
I am getting the "Unfortunately, Crystal Ball has stopped" message. I have retraced steps and not sure why it is now failing. Any help would be greatly appreciated.
Here is the activity_main section:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="top" android:background="@android:color/black" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.crystalball.MainActivity$PlaceholderFragment" >
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball01" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/imageView2"
android:layout_alignLeft="@+id/imageView2"
android:text="Enlighten Me!"
android:textColorLink="#3f0f7f"
android:textSize="24sp"
android:textStyle="bold|italic"
android:typeface="serif" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:weightSum="1" >
<View
android:id="@+id/view1"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="0.2" />
<TextView
android:id="@+id/textView1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.6"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="#3f0f7f"
android:textSize="32sp" />
<View
android:id="@+id/view2"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="0.2" />
</LinearLayout>
</RelativeLayout>
Here is the MainActivity section:
package com.example.crystalball;
import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
private CrystalBall mCrystalBall = new CrystalBall();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
//Declare our View variables and assign them the Views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = (Button) findViewById(R.id.button1);
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String answer = mCrystalBall.getAnAnswer();
//Update the label with our dynamic answer
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
5 Answers
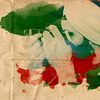
Gunjeet Hattar
14,483 PointsOk here is the issue
The error relates to java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity
The video in treehouse uses the Theme called ** Theme.Black.NoTitleBar.Fullscreen ** . Are you using this?
If yes, then the new android update no longer supports this. However there is a work around as proposed by treehouse
1) Change your project back to using "AppTheme" like it was before in AndroidManifest.xml and the preview.
2) Add the following two lines to the file res/values/styles.xml (inside the <style name="AppTheme"> tag):
<item name="android:windowNoTitle">true</item>
<item name="android:windowFullscreen">true</item>
3) Set the background color to black in the file res/layout/activity_main.xml. The root element, RelativeLayout, is the one that makes up the whole screen. Add this line after the layout_height line but before the closing angle bracket >:
android:background="@android:color/black"
4) Change MainActivity to extend Activity, not ActionBarActivity
Hope this helps. Ask again if you have doubts.
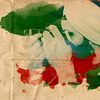
Gunjeet Hattar
14,483 PointsDid you omit the <Relative_Layout*>* tag on purpose or have you not created one at all?
This is how your xml structure should look like
Relative Layout
ImageView
Button
LinearLayout
View1
TextView
View2
Close LinearLayout
Close Relative layout

Robert Shaw
1,567 PointsApologies.....the relative layout got cut off when I pasted it. Thank you for pointing that out.
Here is the complete file.
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="top"
android:background="@android:color/black"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context="com.example.crystalball.MainActivity$PlaceholderFragment" >
<ImageView
android:id="@+id/imageView2"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="fitCenter"
android:src="@drawable/ball01" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/imageView2"
android:layout_alignLeft="@+id/imageView2"
android:text="Enlighten Me!"
android:textColorLink="#3f0f7f"
android:textSize="24sp"
android:textStyle="bold|italic"
android:typeface="serif" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_centerVertical="true"
android:weightSum="1" >
<View
android:id="@+id/view1"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="0.2" />
<TextView
android:id="@+id/textView1"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="0.6"
android:gravity="center_horizontal"
android:shadowColor="@android:color/white"
android:shadowRadius="10"
android:textColor="#3f0f7f"
android:textSize="32sp" />
<View
android:id="@+id/view2"
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_weight="0.2" />
</LinearLayout>
</RelativeLayout>

Robert Shaw
1,567 PointsSorry. For some reason the relative layout will not past....but it is there at the top of the file.
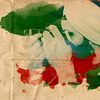
Gunjeet Hattar
14,483 PointsCan you look in the console or the logcat tab and paste the errors here that are generated. It will give a better understanding then.

Robert Shaw
1,567 Points<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="top" android:background="@android:color/black" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.crystalball.MainActivity$PlaceholderFragment" >
LOGCAT
07-17 22:38:31.781: D/AndroidRuntime(1817): Shutting down VM
07-17 22:38:31.801: W/dalvikvm(1817): threadid=1: thread exiting with uncaught exception (group=0xb0d08b20)
07-17 22:38:31.801: E/AndroidRuntime(1817): FATAL EXCEPTION: main
07-17 22:38:31.801: E/AndroidRuntime(1817): Process: com.example.crystalball, PID: 1817
07-17 22:38:31.801: E/AndroidRuntime(1817): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.crystalball/com.example.crystalball.MainActivity}: java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity.
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2195)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2245)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.ActivityThread.access$800(ActivityThread.java:135)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1196)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.os.Handler.dispatchMessage(Handler.java:102)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.os.Looper.loop(Looper.java:136)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.ActivityThread.main(ActivityThread.java:5017)
07-17 22:38:31.801: E/AndroidRuntime(1817): at java.lang.reflect.Method.invokeNative(Native Method)
07-17 22:38:31.801: E/AndroidRuntime(1817): at java.lang.reflect.Method.invoke(Method.java:515)
07-17 22:38:31.801: E/AndroidRuntime(1817): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779)
07-17 22:38:31.801: E/AndroidRuntime(1817): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595)
07-17 22:38:31.801: E/AndroidRuntime(1817): at dalvik.system.NativeStart.main(Native Method)
07-17 22:38:31.801: E/AndroidRuntime(1817): Caused by: java.lang.IllegalStateException: You need to use a Theme.AppCompat theme (or descendant) with this activity.
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.support.v7.app.ActionBarActivityDelegate.onCreate(ActionBarActivityDelegate.java:110)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.support.v7.app.ActionBarActivityDelegateICS.onCreate(ActionBarActivityDelegateICS.java:57)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.support.v7.app.ActionBarActivity.onCreate(ActionBarActivity.java:99)
07-17 22:38:31.801: E/AndroidRuntime(1817): at com.example.crystalball.MainActivity.onCreate(MainActivity.java:17)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.Activity.performCreate(Activity.java:5231)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1087)
07-17 22:38:31.801: E/AndroidRuntime(1817): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2159)
07-17 22:38:31.801: E/AndroidRuntime(1817): ... 11 more
Then I get the Unfortunately Crystal Ball Not Working....message
Thank you.
Endre Juhasz
613 PointsAwesome.
Been looking for an answer for about 2 days, found nothing but retard answers.
Issue solved.
Thank You for taking your time to share this.