Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial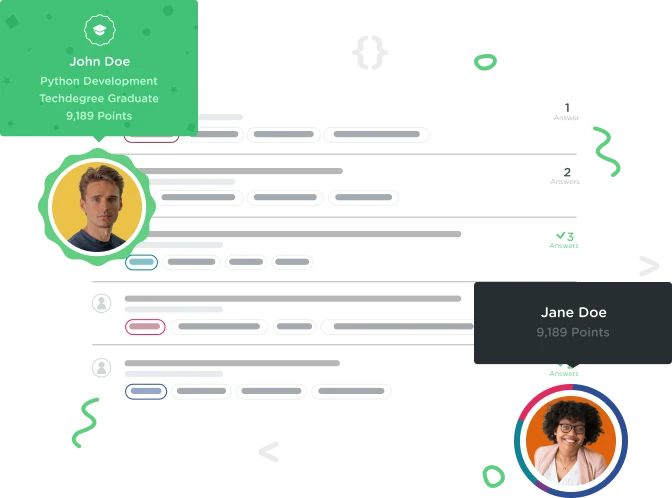
Jacob Safar
890 PointsCrystal Ball Animation(s) not working
I have two problems
when I run the application the Crystal ball does not move (isn't being animated). I don't know what I did wrong or what I am missing.
Now that I am adding an animation for the prediction label (going transparent then back to normal), when I click the app to go to the next prediction the text disappears.
I put my code for the ViewController.h and .m below.
Jacob Safar
890 Points#import <UIKit/UIKit.h>
@class SafarCrystalBall;
@interface SafarViewController : UIViewController
@property (strong, nonatomic) IBOutlet UILabel *predictionLabel;
@property (strong, nonatomic) SafarCrystalBall *crystalBall;
@property (strong, nonatomic) IBOutlet UIImageView *backgroundImageView;
@end
Jacob Safar
890 PointsWhat is wrong with my code :(
Jacob Safar
890 Points@Amit Bijlani can you see what I am doing wrong? I can't move forward until this is resolved :(
2 Answers
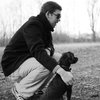
James Carney
16,255 PointsAre you getting an error message or a bug or anything?
Your code looks good at a first glance, did you upload the images to xcode properly?
Jacob Safar
890 PointsNo error messages.
I believe that I did upload them properly.
- I draged and dropped all 160 images into my images.xcassets, then I renamed the Image View CB00001 like Amit did. I also believe that I did the Referencing Outlets (New Referencing Outlet) correctly as you can see in the code above, so I am very confused why these things are not working.
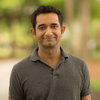
Amit Bijlani
Treehouse Guest TeacherIn your touchesEnded
method you should be calling the makePrediction
method instead you are directly setting the text label.
Jacob Safar
890 PointsJacob Safar
890 Points