Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial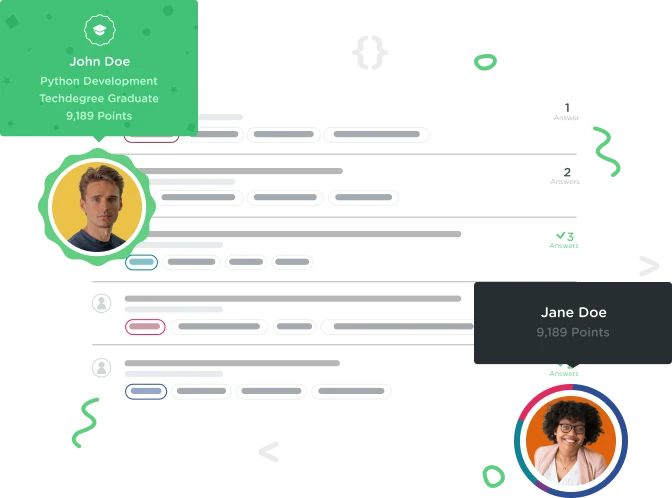

Giuseppe Ienco
1,560 Pointscrystal ball has stopped working.
hello. thanks for taking the time to read this. I do everything in the video 'adding an OnClickListener to a button. but I when I run the emulator. it says the app 'crystal ball' has stopped working. any suggestions?
4 Answers

Nick Edwards
Courses Plus Student 15,766 PointsAh okay, I see what the problem is.
The clues can be seen in the LogCat from the following lines:
E/AndroidRuntime(1114): Caused by: java.lang.NullPointerException 06-10 00:21:14.922: E/AndroidRuntime(1114): at com.example.crystalball.MainActivity.onCreate(MainActivity.java:23)
This tells us the error is a NullPointerException, thrown from MainActivity.java in the method onCreate on line 23. NullPointerExceptions are caused by the following (taken from the Oracle website):
Thrown when an application attempts to use null in a case where an object is required. These include: 1.Calling the instance method of a null object.
- Accessing or modifying the field of a null object.
- Taking the length of null as if it were an array.
- Accessing or modifying the slots of null as if it were an array.
- Throwing null as if it were a Throwable value.
Looking at your code, you've declared the Button variable getAnswerButton as null. This would be fine on its own, except that you've then attached an onclick listener to a null object (getAnswerButton.setOnClickListener
) - hence the null pointer exception. A null object cannot have a method because it's literally an empty object.
So you'll want to declare Button as your View object in the same way as your TextView:
Button getAnswerButton = (Button) findViewById(R.id.button1);
This should solve your problem. I should also point out that it's never a good idea to suppress "null" warnings for exactly this reason - they can break an application very easily if ignored.
Hope this helps!

Nick Edwards
Courses Plus Student 15,766 PointsHi Giusweppe,
Could you post the contents of LogCat (there should be a 'LogCat' tab at the bottom of Eclipse which displays the error) here along with your MainActivity.java and activity_main.xml?
Don't forget to use the Markdown formatting below so the code is easier to read :)

Giuseppe Ienco
1,560 Points- LogCat
. 06-10 00:21:14.902: D/AndroidRuntime(1114): Shutting down VM 06-10 00:21:14.902: W/dalvikvm(1114): threadid=1: thread exiting with uncaught exception (group=0xb1b0fba8) 06-10 00:21:14.922: E/AndroidRuntime(1114): FATAL EXCEPTION: main 06-10 00:21:14.922: E/AndroidRuntime(1114): Process: com.example.crystalball, PID: 1114 06-10 00:21:14.922: E/AndroidRuntime(1114): java.lang.RuntimeException: Unable to start activity ComponentInfo{com.example.crystalball/com.example.crystalball.MainActivity}: java.lang.NullPointerException 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2195) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.ActivityThread.handleLaunchActivity(ActivityThread.java:2245) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.ActivityThread.access$800(ActivityThread.java:135) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.ActivityThread$H.handleMessage(ActivityThread.java:1196) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.os.Handler.dispatchMessage(Handler.java:102) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.os.Looper.loop(Looper.java:136) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.ActivityThread.main(ActivityThread.java:5017) 06-10 00:21:14.922: E/AndroidRuntime(1114): at java.lang.reflect.Method.invokeNative(Native Method) 06-10 00:21:14.922: E/AndroidRuntime(1114): at java.lang.reflect.Method.invoke(Method.java:515) 06-10 00:21:14.922: E/AndroidRuntime(1114): at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:779) 06-10 00:21:14.922: E/AndroidRuntime(1114): at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:595) 06-10 00:21:14.922: E/AndroidRuntime(1114): at dalvik.system.NativeStart.main(Native Method) 06-10 00:21:14.922: E/AndroidRuntime(1114): Caused by: java.lang.NullPointerException 06-10 00:21:14.922: E/AndroidRuntime(1114): at com.example.crystalball.MainActivity.onCreate(MainActivity.java:23) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.Activity.performCreate(Activity.java:5231) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.Instrumentation.callActivityOnCreate(Instrumentation.java:1087) 06-10 00:21:14.922: E/AndroidRuntime(1114): at android.app.ActivityThread.performLaunchActivity(ActivityThread.java:2159)
- ActivityMain.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.crystalball.MainActivity$PlaceholderFragment" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true"
android:textSize="32sp" />
<Button
android:id="@+id/button1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_below="@+id/textView1"
android:layout_centerHorizontal="true"
android:text="Enlighten Me!" />
</RelativeLayout>
- MainActivity.java
package com.example.crystalball;
import android.os.Bundle; import android.support.v7.app.ActionBarActivity; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.widget.Button; import android.widget.TextView;
public class MainActivity extends ActionBarActivity {
@SuppressWarnings("null")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Declare Our View Variables and assign them the views from the layout file
final TextView answerLabel = (TextView) findViewById(R.id.textView1);
Button getAnswerButton = null;
getAnswerButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// The Button was Clicked, so update the answer label with an answer
String answer = "Yes";
answerLabel.setText(answer);
}
});
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
I hope that is what you meant by markdown. thank you for taking the time to help :) i followed the instructions step by step. dont know where im going wrong.

Giuseppe Ienco
1,560 Pointsthankyou so much for helping me :) i understand what you mean by null now :)

Nick Edwards
Courses Plus Student 15,766 PointsNo problem, glad to help!