Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial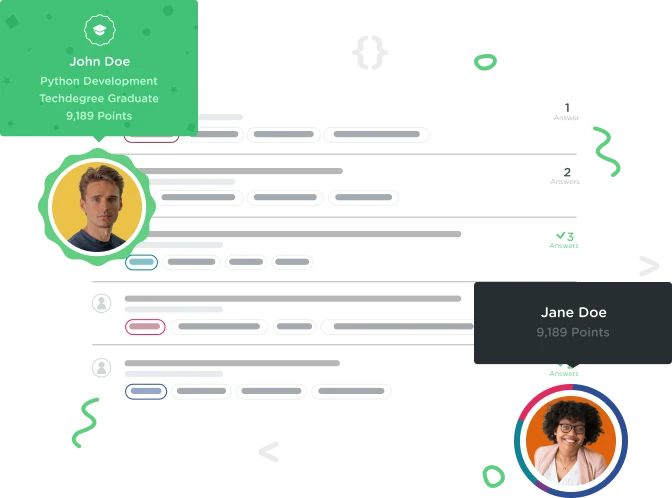
Rodrigo Chousal
16,009 PointsCrystallBall App Challenges
My code works, but I really have no idea why... I'm getting a warning (not an error, the program runs exactly as expected) that reads: Incompatible pointer types assigning to' UIColor *' from 'NSString *'
My code is in the comments!
Rodrigo Chousal
16,009 PointsRCViewController.m:
#import "RCViewController.h"
#import "RCCrystalBall.h"
@interface RCViewController ()
@end
@implementation RCViewController
- (void)viewDidLoad
{
[super viewDidLoad];
self.crystalBall = [[RCCrystalBall alloc] init];
}
- (void)didReceiveMemoryWarning
{
[super didReceiveMemoryWarning];
// Dispose of any resources that can be recreated.
}
- (IBAction)buttonPressed {
self.predictionLabel.text = [self.crystalBall randomPrediction];
self.predictionLabel.textColor = [self.crystalBall randomColors];
NSLog(@"Button Pressed\n");
}
@end
Rodrigo Chousal
16,009 PointsRCCrystalBall.h:
#import <Foundation/Foundation.h>
@interface RCCrystalBall : NSObject{
NSArray *_predictions;
}
@property (strong, nonatomic, readonly) NSArray *predictions;
@property (strong, nonatomic) NSArray *colors;
- (NSString *) randomPrediction;
- (NSString *) randomColors;
@end
Rodrigo Chousal
16,009 PointsRCCrystalBall.m:
#import "RCCrystalBall.h"
@implementation RCCrystalBall
- (NSArray *) predictions {
if (_predictions == nil) {
_predictions = [[NSArray alloc] initWithObjects:@"It is certain", @"It is decidedly so", @"All signs say yes", @"The stars are not aligned", @"My reply is no", @"It is doubtful", @"Better not tell you now", @"Concentrate and ask again", @"Unable to answer now", @"Just do it", @"You will endure", @"All signs say no", @"The stars are aligned", @"My reply is yes", @"It is uncertain", @"Better tell you now", @"Don't do it", @"You will not endure", nil];
}
return _predictions;
}
- (NSString *) randomPrediction {
int random = arc4random_uniform(self.predictions.count);
return [self.predictions objectAtIndex:random];
}
- (NSArray *) colors {
if (_colors == nil) {
_colors = [[NSArray alloc] initWithObjects:[UIColor blackColor], [UIColor darkGrayColor], [UIColor lightGrayColor], [UIColor whiteColor], [UIColor grayColor], [UIColor redColor], [UIColor greenColor], [UIColor blueColor], [UIColor cyanColor], [UIColor yellowColor], [UIColor magentaColor], [UIColor orangeColor], [UIColor purpleColor], [UIColor brownColor], nil];
}
return _colors;
}
- (NSString *) randomColors {
int randomColor = arc4random_uniform(self.colors.count);
return [self.colors objectAtIndex:randomColor];
}
@end
``
1 Answer

Stone Preston
42,016 Pointsyour return type is wrong on your randomColors method
- (NSString *) randomColors {
int randomColor = arc4random_uniform(self.colors.count);
return [self.colors objectAtIndex:randomColor];
}
since your colors array contains UIColor objects, you need to return a UIColor, not an NSString
- (UIColor *) randomColors {
int randomColor = arc4random_uniform(self.colors.count);
return [self.colors objectAtIndex:randomColor];
}
Rodrigo Chousal
16,009 PointsThank you for such a quick response!

Stone Preston
42,016 Pointsno problem : )
Rodrigo Chousal
16,009 PointsRodrigo Chousal
16,009 PointsRCViewController.h: