Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial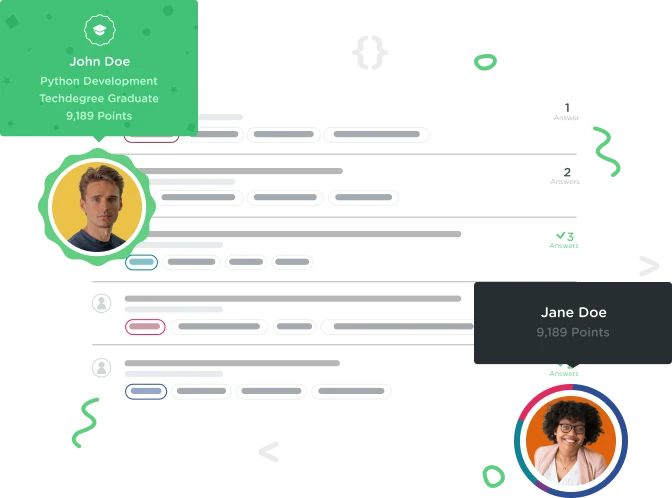

Christopher Aberly
1,241 PointsCS1501: No overload for method takes '1' arguments.
I'm getting a CS1501 error on my DistanceTo method after writing the code associated with this video. I tried a few things, but I'm not exactly sure what I'm missing here everything looks to be the same as the videos for the "Point" file and the "MapLocation" file. I'm assuming that I'm not passing the right number of arguments into the method, but I can't figure out how many I'm actually passing to see what might be the problem.
I noticed that the MapLocation Object has 3 parameters (x, y, and map) while the DistanceTo takes only 2 (x,y), but the code in the video didn't mention modifications to this, so I'm assuming that this is ok.
Below is my code in the MapLocation file
namespace TreehouseDefense {
class MapLocation : Point {
public MapLocation(int x, int y, Map map) : base (x, y)
{
if (!map.OnMap(this))
{
throw new OutOfBoundsException(x + "," + y + " is outside the boundaries of the map!");
}
}
public bool InRangeOf(MapLocation location, int range)
{
return DistanceTo(location) <= range;
}
}
}
For Point File (object)
using System;
namespace TreehouseDefense
{ class Point { public readonly int X; public readonly int Y;
public Point(int x, int y)
{
X = x;
Y = y;
}
public int DistanceTo(int x, int y)
{
return (int)Math.Sqrt(Math.Pow(X - x , 2) + Math.Pow(Y - y , 2));
}
} }
2 Answers
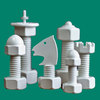
Steven Parker
231,275 PointsAs you observed, "DistanceTo" is defined to take 2 arguments. But it is being called with just one argument ("DistanceTo(location)
") in the "InRangeOf" method.
You could change the call to pass in two arguments, but I seem to recall that the project eventually has a 1-argument overload. So if you didn't miss something, you could just be in an intermediate point right before the overload gets added.
UPDATE: In the Overloading Methods video back in stage 1, the overload of "DistanceTo" was created in the "Point" object. The original takes 2 int arguments, but the new one takes a "Point" (which can also be a "MapLocation").

Christopher Aberly
1,241 PointsI've made it through the whole course and gone back through all of the videos to figure out what I've done wrong and can't find it. It looks like the location variable being passed into the DistanceTo method is of MapLocation type, which is an "x", "y", and "map" value. I'm not sure what is going on here, but I've written exactly what is in the videos and its not passing through. I don't recall anything about an override?!
So, to check to see if the additional "map" parameter was causing the issue I changed the type to a "Point"
I tried changing the location type to a Point (from a different class) which definitely only has two input arguments. Note, this is the same class that the "DistanceTo" method is initiated in and on. No dice. Same error.
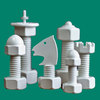
Steven Parker
231,275 PointsIt does seem that you missed a bit of a video. See the update I added to my answer.