Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial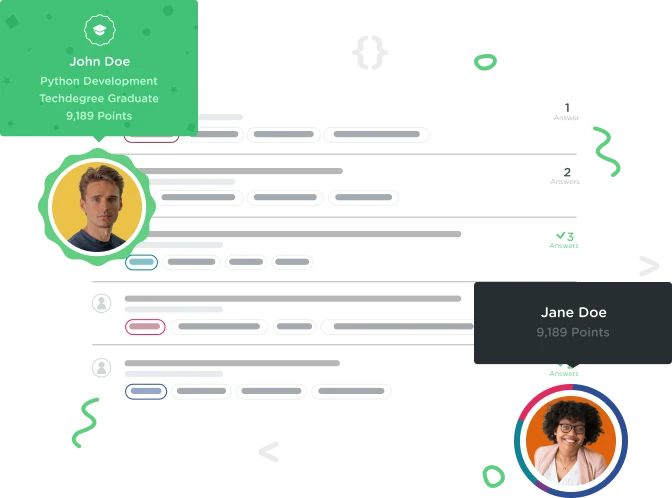
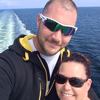
Shawn Wilson
iOS Development Techdegree Student 7,049 PointsCurious to know if my solution is considered up to PAR?
just looking for a critique on my code for this project. the Solution works and I know thats what counts here but i learn better with constructive criticism if you wanna look this over and provide some feedback, tips or hints that would be great!
Thanks all,
/* ********************** */
/* First Random Generator */
/* ********************** */
alert("This number generator will randomly generate a number with the numbers you provide.");
var num1 = prompt("Please select your first number");
var num1 = parseInt(num1);
var random = Math.floor(Math.random() * num1 + 1);
document.write("Your first random number is: " + random + " ");
/* ************************ */
/* Second Random Generator */
/* ************************ */
var num2 = prompt("Please enter your first number");
var num2 = parseInt(num2);
var num3 = prompt("Please enter your second number");
var num3 = parseInt(num3);
var random2 = Math.ceil(Math.random() * (num2 - num3)) + num1;
document.write("Your second random number is: " + random2);
alert("Thanks for messing around with this little project.");
3 Answers
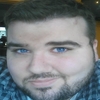
Marcus Parsons
15,719 PointsHey Shawn Wilson,
In the first and second number generators, you can be more efficient by combining the parseInt function with the prompt like so:
//Gets the base 10 integer of a prompt
var num1 = parseInt(prompt("Please select your first number"), 10);
In your second number generator, the code just isn't quite right. The point of the 2nd generator is to generate a number that is inclusive of a given range. In order to do that, you have to follow certain mathematical procedures. With your code, the numbers would be all over the place. But, no worries, here is a look at what your code should resemble:
var randMin = parseInt(prompt("Enter a minimum value for your random number:"), 10);
var randMax = parseInt(prompt("Enter a maximum value for your random number:"), 10);
var randNumber = Math.floor(Math.random() * (randMax - randMin + 1)) + randMin;
//For browsers with a Web Console i.e. Firefox or Chrome
console.log("Your random number is: " + randNumber);
//Other browsers
//alert("Your random number is: " + randNumber);
Now when you run that above code, you will always get a number that is within the range provided: [randMin, randMax] inclusive. I hope that helps!
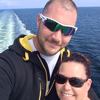
Shawn Wilson
iOS Development Techdegree Student 7,049 Pointsit sure dose! as soon as i looked at your code, I saw what you were talking about and it clicked me into the ah-ha moment.
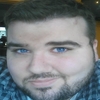
Marcus Parsons
15,719 PointsAwesome! I just like to be very thorough in my explanations haha Good luck, Shawn!

Gina Bégin
Courses Plus Student 8,613 PointsHey Marcus Parsons — sorry to bring up an older discussion, but I'm on this challenge and having a little trouble. I liked your explanation, so I went to try the code you provided; however, I couldn't get it to go past providing my max number — that was the last alert that showed up. A console didn't pop up and no message showed up on the main web page for me (running Chrome). Was there supposed to be a document.write in the code by chance? Or is my browser just faulty?
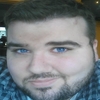
Marcus Parsons
15,719 PointsHi Gina,
If you go into your console, you'll see the command. Notice that I used console.log
which logs information out to the console in Chrome and Firefox (and I believe Safari). The console doesn't pop up when you use it; you have to manually bring it up to see things in your console.
You can comment out the line that uses console.log
and then uncomment the line for the alert
which shows a pop up. I don't like alerts because they can be annoying if used too often.
var randMin = parseInt(prompt("Enter a minimum value for your random number:"), 10);
var randMax = parseInt(prompt("Enter a maximum value for your random number:"), 10);
var randNumber = Math.floor(Math.random() * (randMax - randMin + 1)) + randMin;
//For browsers with a Web Console i.e. Firefox or Chrome
//console.log("Your random number is: " + randNumber);
//Other browsers
alert("Your random number is: " + randNumber);
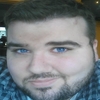
Marcus Parsons
15,719 PointsAnother problem with using alerts is that when you start writing loops that can go on for an indeterminate amount of time (i.e. a while or do while loop), alert
boxes can cause the page to become completely unresponsive because you can't close any tabs while an alert box is being shown. Luckily for us, modern browsers give us the option to stop the page from displaying these boxes after more than one is shown. It can become a giant mess if used improperly.
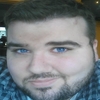
Marcus Parsons
15,719 PointsAnd one more thing haha Avoid using document.write
except with testing a piece of basic code. document.write
is a terrible command to use in applications because it overwrites all of your DOM structure in your page with the first value you pass into it. Every proceeding value passed into it is added to the first one. So, let's say you set up this nice form for people to use with text boxes, a textarea, some select options, etc. and then you use document.write
to output some information to the page, it literally overwrites all of that information such that it no longer exists on the page.
If you want to output text to a page, it's best to target an element and use that element'stextContent
property or innerHTML
when inserting elements, as well.
Okay that's it :)
Shawn Wilson
iOS Development Techdegree Student 7,049 PointsShawn Wilson
iOS Development Techdegree Student 7,049 PointsThanks for the feedback! Great comments ill try your methods and keep it in my head moving forward!
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsAnytime, Shawn! Just in case you were wondering about the math involved there:
Let's say we get two numbers in: 50 and 100. That's our minimum and maximum value. If we do the math for
randNum
you can see that the number will always be within that range, inclusive.Math.random() has a minimum possible value of 0 and a maximum possible value of 0.9999....
Minimum
So, taking a look at the minimum possible value for
randNum
, let's plug in the values:Math.random() rolls to 0.
Math.floor(0 * (100-50+1)) + 50.
0 * anything is 0, so:
0 + 50 = 50
Maximum
And taking a look at the maximum possible value for
randNum
:Math.random() rolls to 0.9999...
Math.floor(0.9999... * (100-50+1)) + 50.
Math.floor(0.9999... * 51) + 50.
Math.floor(50.9949) + 50.
50 + 50 = 100
So, now you can see that the range will always be inclusive of whatever the minimum and maximum numbers given. Does that make a little bit more clear, Shawn?